一、字符串操作: 1.从键盘上输入一个字符串,并显示到屏幕上 2、统计字符串中数字的个数。统计字符串中小字母‘a’的个数,并把‘a’替换成‘A’ 3、从键盘上输入另一个字符串,将两个字符串进行拼接 4、在字符串中查找子串“is”,并返回第一个查找的位置 5、将拼接好的字符串逆序输出
时间: 2024-02-16 18:02:52 浏览: 69
好的,我来回答你的问题。
1. 以下是C++代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
cout << "请输入一个字符串:";
getline(cin, str);
cout << "你输入的字符串是:" << str << endl;
return 0;
}
```
2. 统计字符串中数字的个数和小字母'a'的个数,并把'a'替换成'A',以下是C++代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
cout << "请输入一个字符串:";
getline(cin, str);
int digit_count = 0;
int a_count = 0;
for (int i = 0; i < str.length(); i++) {
if (isdigit(str[i])) {
digit_count++;
}
if (str[i] == 'a') {
a_count++;
str[i] = 'A';
}
}
cout << "数字个数:" << digit_count << endl;
cout << "小写字母a个数:" << a_count << endl;
cout << "替换后的字符串:" << str << endl;
return 0;
}
```
3. 从键盘上输入另一个字符串,将两个字符串进行拼接,以下是C++代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1, str2;
cout << "请输入第一个字符串:";
getline(cin, str1);
cout << "请输入第二个字符串:";
getline(cin, str2);
string result = str1 + str2;
cout << "拼接后的字符串:" << result << endl;
return 0;
}
```
4. 在字符串中查找子串“is”,并返回第一个查找的位置,以下是C++代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
cout << "请输入一个字符串:";
getline(cin, str);
size_t pos = str.find("is");
if (pos != string::npos) {
cout << "第一次出现的位置:" << pos << endl;
} else {
cout << "未找到子串" << endl;
}
return 0;
}
```
5. 将拼接好的字符串逆序输出,以下是C++代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1, str2;
cout << "请输入第一个字符串:";
getline(cin, str1);
cout << "请输入第二个字符串:";
getline(cin, str2);
string result = str1 + str2;
cout << "拼接后的字符串:" << result << endl;
cout << "逆序输出字符串:";
for (int i = result.length() - 1; i >= 0; i--) {
cout << result[i];
}
cout << endl;
return 0;
}
```
希望我的回答能够帮助到你!
阅读全文
相关推荐
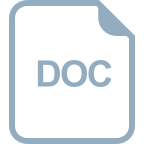
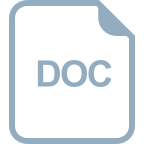
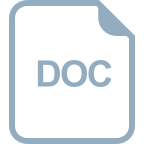



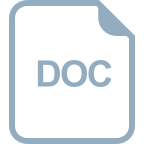
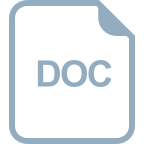
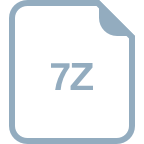
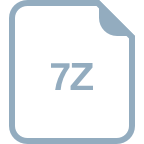