for n = nmin:2:nmax
时间: 2024-02-09 10:08:15 浏览: 31
The syntax "for n = nmin:2:nmax" is a loop in MATLAB that iterates over the values of "n" starting from "nmin" and incrementing by 2 until it reaches or exceeds "nmax".
During each iteration of the loop, the value of "n" is updated and the code within the loop is executed. This loop is typically used when you want to perform the same set of operations on a range of values of "n".
Here's an example of how you can use this loop to print the values of "n" from 1 to 10, incrementing by 2:
```
nmin = 1;
nmax = 10;
for n = nmin:2:nmax
disp(n);
end
```
This will output:
```
1
3
5
7
9
```
I hope this helps! Let me know if you have any further questions.
相关问题
5114人阅读\n#include\n\nmain()\n\n{\n\nint i,a,b;\n\nint max,min;\n\nint max=0,min=0;\n\nprintf(\"please input 10 numbers:\");\n\nscanf(\"%d\",&a);\n\nmax = a;\n\nmin = a;\n\nfor(i=2;i\u003C=10;i++)\n\n{\n\nscanf(\"%d\",&a);\n\nif(a>max)\n\nmax=a;\n\nif(b\n\nmin=a;\n\n}\n\nprintf(\"max=%d\\n,max);\n\n}
这段代码存在一些问题,可能是因为复制粘贴出现了格式问题,下面是一个修复后的版本:
```
#include <stdio.h>
int main()
{
int i,a,max,min;
printf("Please input 10 numbers:\n");
scanf("%d",&a);
max = a;
min = a;
for(i=2;i<=10;i++)
{
scanf("%d",&a);
if(a>max)
max=a;
if(a<min)
min=a;
}
printf("Max=%d, Min=%d\n", max, min);
return 0;
}
```
这段代码的作用是输入 10 个数字,然后输出其中的最大值和最小值。
#include<stdio.h> #include<stdlib.h> int main() { int n; scanf("%d",&n); float *p; p=(float*)malloc(sizeof(float)*n); for(int i=0;i<n;i++) { scanf("%f",&p[i]); } float average=0,sum=0; int min=0,max=0; for(int i=0;i<n;i++) { sum+=p[i]; } average=sum/n; for(int i=0;i<n;i++) { if(p[i]<p[min]) { min=i; } } for(int i=0;i<n;i++) { if(p[i]>p[max]) { max=i; } } printf("average = %.2f\nmax = %.2f\nmin = %.2f",average,p[max],p[min]); return 0; }将它转化为python语言
以下是将该 C 语言代码转化为 Python 语言的示例代码:
```python
n = int(input())
p = []
for i in range(n):
p.append(float(input()))
average = sum(p) / n
min_index = p.index(min(p))
max_index = p.index(max(p))
print("average = %.2f" % average)
print("max = %.2f" % p[max_index])
print("min = %.2f" % p[min_index])
```
这段 Python 代码的功能与上面的 C 语言代码相同,都是读入 n 个浮点数,计算它们的平均值、最大值和最小值,并输出这些值。不同之处在于 Python 中不需要显式地调用 `malloc()` 分配内存,而是使用列表来存储浮点数。另外,在 Python 中字符串格式化的方式也有所不同。
阅读全文
相关推荐
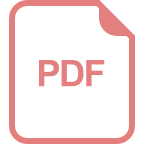


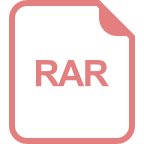
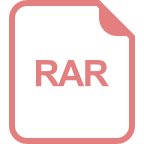
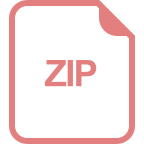
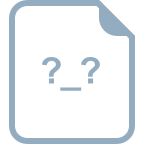
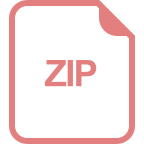
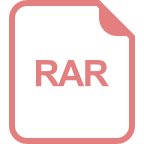
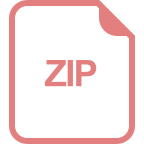
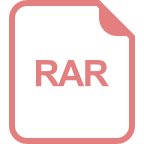