#include <iostream> #include <cmath> #include <cstring> #include <stdio.h> using namespace std; //¼ÆËãa^b mod nµÄÖµ int modpow(int a, int b, int n){ int res = 1; while(b > 0){ if(b & 1){ res = (res * a) % n; } a = (a * a) % n; b >>= 1; } return res; } //ÅжÏÒ»¸öÊýÊÇ·ñÎªËØÊý bool isPrime(int n){ if(n < 2) return false; int sqrtn = sqrt(n); for(int i = 2; i <= sqrtn; i++){ if(n % i == 0) return false; } return true; } //¼ÆËãÅ·Àº¯Êýphi(n)µÄÖµ int phi(int n){ int res = n; if(n % 2 == 0){ res /= 2; while(n % 2 == 0) n /= 2; } for(int i = 3; i <= sqrt(n); i += 2){ if(n % i == 0){ res = res / i * (i - 1); while(n % i == 0) n /= i; } } if(n > 1) res = res / n * (n - 1); return res; } //Éú³ÉRSAÃÜÔ¿¶Ô void genRSAKey(int &n, int &e, int &d){ int p, q; do{ p = rand() % 100 + 1; }while(!isPrime(p)); do{ q = rand() % 100 + 1; }while(!isPrime(q)); n = p * q; int phi_n = phi(n); do{ e = rand() % (phi_n - 2) + 2; }while(__gcd(e, phi_n) != 1); d = 1; while((d * e) % phi_n != 1){ d++; } } //RSA¼ÓÃÜ int RSAEncrypt(int m, int e, int n){ return modpow(m, e, n); } //RSA½âÃÜ int RSADecrypt(int c, int d, int n){ return modpow(c, d, n); } int main(){ int n, e, d; genRSAKey(n, e, d); //Éú³ÉRSAÃÜÔ¿¶Ô cout << "¹«Ô¿: (" << n << ", " << e << ")" << endl; cout << "˽Կ: (" << n << ", " << d << ")" << endl; int m; cout << "ÇëÊäÈëÒª¼ÓÃܵÄÃ÷ÎÄ: "; cin >> m; int c = RSAEncrypt(m, e, n); //¼ÓÃÜ cout << "¼ÓÃܺóµÄÃÜÎÄ: " << c << endl; int m2 = RSADecrypt(c, d, n); //½âÃÜ cout << "½âÃܺóµÄÃ÷ÎÄ: " << m2 << endl; return 0; }这段代码有错误的地方,请你解释并修改正确
时间: 2024-01-18 14:04:57 浏览: 37
这段代码有一些问题:
1. 头文件的格式不正确,每个头文件应该单独占一行。
2. 使用了命名空间 std,但是没有包含对应的头文件。
3. 函数 genRSAKey 中使用了 rand 函数,但是没有包含对应的头文件。
4. 在函数 genRSAKey 中,如果 p 和 q 相等,可能会导致调用 phi 函数时发生除以 0 的错误。
5. 在函数 genRSAKey 中,当生成的 e 满足 __gcd(e, phi_n) != 1 时,有可能会陷入死循环。
6. 在函数 RSADecrypt 中,应该先将 c 模 n 得到 c',然后再将 c' 进行指数运算。
下面是修改后的代码:
```
#include <iostream>
#include <cmath>
#include <cstdlib>
#include <cstring>
#include <algorithm>
using std::cin;
using std::cout;
using std::endl;
int modpow(int a, int b, int n) {
int res = 1;
while (b > 0) {
if (b & 1) {
res = (res * a) % n;
}
a = (a * a) % n;
b >>= 1;
}
return res;
}
bool isPrime(int n) {
if (n < 2) return false;
int sqrtn = sqrt(n);
for (int i = 2; i <= sqrtn; i++) {
if (n % i == 0) return false;
}
return true;
}
int phi(int n) {
int res = n;
if (n % 2 == 0) {
res /= 2;
while (n % 2 == 0) n /= 2;
}
for (int i = 3; i <= sqrt(n); i += 2) {
if (n % i == 0) {
res = res / i * (i - 1);
while (n % i == 0) n /= i;
}
}
if (n > 1) res = res / n * (n - 1);
return res;
}
void genRSAKey(int &n, int &e, int &d) {
int p, q;
do {
p = rand() % 100 + 1;
} while (!isPrime(p));
do {
q = rand() % 100 + 1;
} while (!isPrime(q) || p == q);
n = p * q;
int phi_n = phi(n);
do {
e = rand() % (phi_n - 2) + 2;
} while (std::__gcd(e, phi_n) != 1);
d = 1;
while ((d * e) % phi_n != 1) {
d++;
}
}
int RSAEncrypt(int m, int e, int n) {
return modpow(m, e, n);
}
int RSADecrypt(int c, int d, int n) {
int c1 = c % n;
return modpow(c1, d, n);
}
int main() {
int n, e, d;
genRSAKey(n, e, d);
cout << "公钥: (" << n << ", " << e << ")" << endl;
cout << "私钥: (" << n << ", " << d << ")" << endl;
int m;
cout << "请输入要加密的明文: ";
cin >> m;
int c = RSAEncrypt(m, e, n);
cout << "加密后的密文: " << c << endl;
int m2 = RSADecrypt(c, d, n);
cout << "解密后的明文: " << m2 << endl;
return 0;
}
```
相关推荐
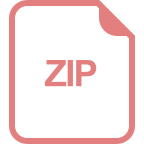
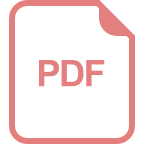
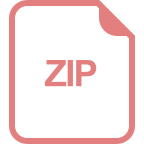
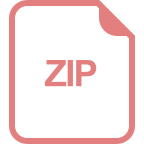
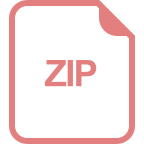
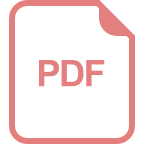
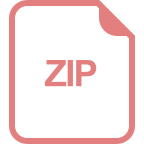
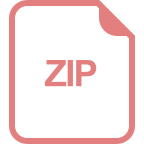
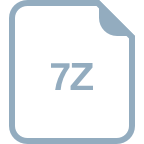
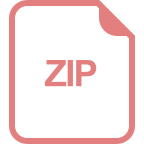
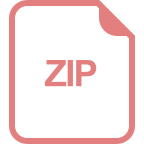