写一个python接口测试类发给我
时间: 2023-09-03 09:27:16 浏览: 147
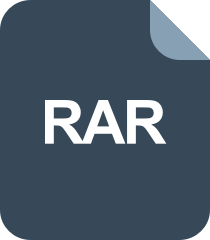
python接口测试代码
### 回答1:
我可以给你一个例子,看看这个python接口测试类是否对你有用:class TestInterface:
def __init__(self, url):
self.url = url
def test_get_method(self):
r = requests.get(self.url)
return r
def test_post_method(self, params):
r = requests.post(self.url, params)
return r
### 回答2:
以下是一个简单的Python接口测试类的示例代码:
```python
import requests
class ApiTest:
def __init__(self, base_url):
self.base_url = base_url
def get_request(self, endpoint, params=None):
url = self.base_url + endpoint
response = requests.get(url, params=params)
return response.json()
def post_request(self, endpoint, data=None):
url = self.base_url + endpoint
response = requests.post(url, json=data)
return response.json()
def put_request(self, endpoint, data=None):
url = self.base_url + endpoint
response = requests.put(url, json=data)
return response.json()
def delete_request(self, endpoint):
url = self.base_url + endpoint
response = requests.delete(url)
return response.json()
# 示例用法
api = ApiTest("http://example.com/api/")
response = api.get_request("users/1")
print(response)
```
以上是一个基本的接口测试类,可以通过传入基本的URL来进行接口请求。类中包含了常用的GET、POST、PUT和DELETE方法,分别用于发送不同类型的接口请求,并返回响应结果。
示例中的`get_request`方法用于发送GET请求,`post_request`方法用于发送POST请求,`put_request`方法用于发送PUT请求,`delete_request`方法用于发送DELETE请求。你可以根据具体的接口需求修改和扩展这些方法。
此外,示例代码中使用了第三方库`requests`来方便地发送HTTP请求和获取响应结果。请确保你的Python环境中已安装该库。
你可以根据需要调用适当的方法并传入相应的参数来发起接口请求,并处理返回的响应结果。在示例中,我们发送了一个GET请求,并打印出响应结果。
注意:为了让上述示例正常工作,你需要将`base_url`参数替换为你要测试的实际接口的基础URL。
### 回答3:
当然,我可以为您编写一个简单的Python接口测试类。那么我们先来定义一个名为 `InterfaceTest` 的类,包含以下方法:
```python
import requests
class InterfaceTest:
def __init__(self, url):
self.url = url
def send_get_request(self, params=None):
response = requests.get(self.url, params=params)
return response.json()
def send_post_request(self, data=None):
response = requests.post(self.url, json=data)
return response.json()
```
以上代码定义了一个名为 `InterfaceTest` 的类,该类接收一个指定的URL作为参数。
接下来,我们定义了两个方法: `send_get_request` 和 `send_post_request`。方法中使用了 `requests` 库来发送GET和POST请求,它们分别接收一个可选的参数来传递请求的参数或数据。这些方法将返回响应的JSON数据。
例如,假设我们要测试一个接口 `https://api.example.com/users`,我们可以创建一个 `InterfaceTest` 对象并调用相应的方法来发送请求。下面是一个简单的示例:
```python
# 创建一个InterfaceTest对象,并指定测试接口的URL
test = InterfaceTest("https://api.example.com/users")
# 发送GET请求
response = test.send_get_request()
print(response)
# 发送POST请求
data = {"name": "John", "age": 30}
response = test.send_post_request(data)
print(response)
```
通过以上代码,我们可以发送GET和POST请求,并打印响应的JSON数据。
请注意,以上代码只是一个简单的示例,具体根据您实际的接口和需求进行调整。希望能对您有帮助!
阅读全文
相关推荐
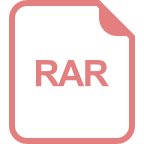
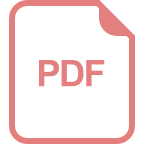
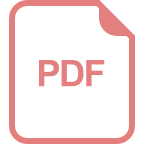
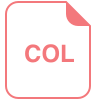
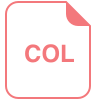
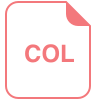
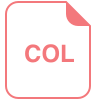
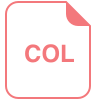
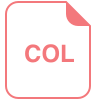
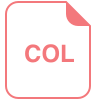
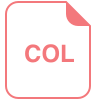
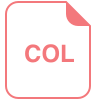
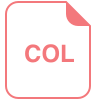
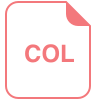