展示一个python发送html电子邮件 邮件正文包括图片和地址链接的实例
时间: 2024-10-24 16:07:44 浏览: 19
在Python中,你可以使用smtplib和email库来发送HTML格式的带图片和链接的电子邮件。这里是一个基本的示例:
```python
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.image import MIMEImage
# 设置邮件内容
def create_email(subject, html_content, sender, recipient, password):
msg = MIMEMultipart('alternative')
msg['Subject'] = subject
msg['From'] = sender
msg['To'] = recipient
# 添加HTML部分
part1 = MIMEText(html_content, 'html')
msg.attach(part1)
# 添加图片
with open('image.jpg', 'rb') as img_file:
image = MIMEImage(img_file.read())
image.add_header('Content-Disposition', 'attachment', filename='image.jpg')
msg.attach(image)
# 发送邮件
try:
server = smtplib.SMTP_SSL('smtp.gmail.com', 465)
server.login(sender, password)
server.sendmail(sender, recipient, msg.as_string())
server.quit()
print("Email sent successfully.")
except Exception as e:
print(f"Error sending email: {str(e)}")
# 使用函数
subject = "Test Email with Image and Link"
sender = "your-email@gmail.com"
recipient = "receiver-email@example.com"
password = "your-password"
html_content = """
<html>
<body>
<h1>Hello World</h1>
<a href="https://example.com">Visit Example Website</a>
<img src="cid:image">
</body>
</html>
"""
create_email(subject, html_content, sender, recipient, password)
```
在这个例子中,你需要替换`sender`, `recipient`, 和 `password`为你自己的邮箱信息。注意,如果你的SMTP服务器不是Gmail,那么需要将`'smtp.gmail.com'`改为相应的SMTP服务器地址。
阅读全文
相关推荐
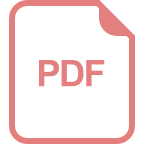
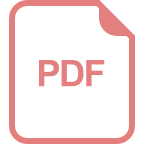
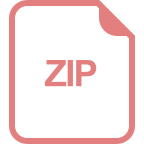
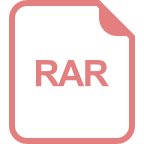
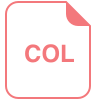
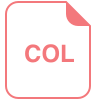
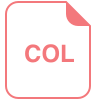
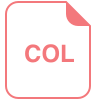
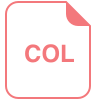
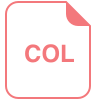
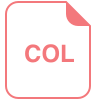
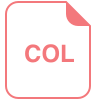
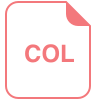
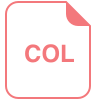
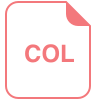
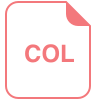
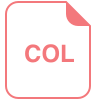
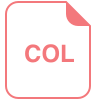

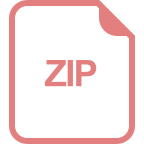