帮我用java写一个读取excel美航数据的方法
时间: 2024-05-10 22:20:01 浏览: 18
以下是一个读取Excel美航数据的Java方法的示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ReadExcelData {
public static List<String[]> readExcelFile(String filePath) {
List<String[]> data = new ArrayList<String[]>();
FileInputStream inputStream = null;
Workbook workbook = null;
try {
File file = new File(filePath);
inputStream = new FileInputStream(file);
// Create Workbook instance holding reference to .xlsx file
workbook = new XSSFWorkbook(inputStream);
// Get first/desired sheet from the workbook
Sheet sheet = workbook.getSheetAt(0);
// Iterate through each rows one by one
Iterator<Row> rowIterator = sheet.iterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
String[] rowData = new String[7];
// For each row, iterate through all the columns
Iterator<Cell> cellIterator = row.cellIterator();
int i = 0;
while (cellIterator.hasNext()) {
Cell cell = cellIterator.next();
// Check the cell type and format accordingly
switch (cell.getCellType()) {
case Cell.CELL_TYPE_NUMERIC:
rowData[i] = String.valueOf(cell.getNumericCellValue());
break;
case Cell.CELL_TYPE_STRING:
rowData[i] = cell.getStringCellValue();
break;
}
i++;
}
data.add(rowData);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (workbook != null) {
workbook.close();
}
if (inputStream != null) {
inputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return data;
}
public static void main(String[] args) {
String filePath = "C:\\Users\\user\\Desktop\\American-Airlines.xlsx";
List<String[]> data = readExcelFile(filePath);
for (String[] rowData : data) {
for (String cellData : rowData) {
System.out.print(cellData + "\t");
}
System.out.println();
}
}
}
```
该方法使用Apache POI库来读取Excel文件。在这个例子中,我们假设Excel文件在本地计算机上的路径为"C:\Users\user\Desktop\American-Airlines.xlsx"。该方法将Excel文件的数据读取到一个字符串数组列表中,每个数组表示Excel文件中的一行数据。在main()方法中,我们遍历这个列表并打印出每一行数据。
相关推荐
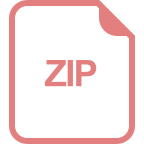
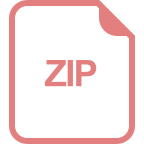
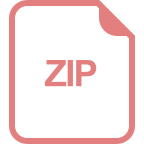
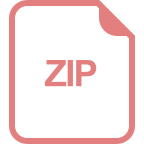
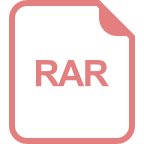
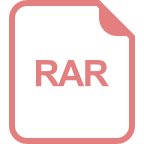
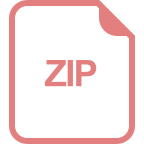
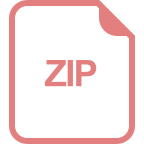
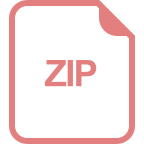
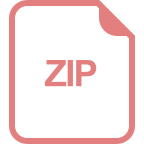
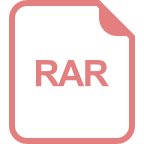
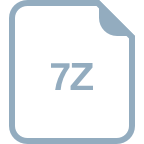
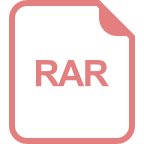
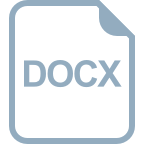
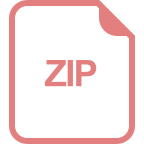
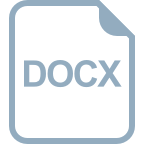