如何用Python的数组来统计一个字符串中每个字符出现的次数
时间: 2024-03-03 07:49:03 浏览: 21
可以使用Python中的字典来实现这个功能。具体步骤如下:
1. 定义一个空字典,用于存储每个字符出现的次数。
2. 遍历字符串中的每个字符,如果该字符已经在字典中存在,则将对应的计数器加1;否则将该字符添加到字典中,并将计数器初始化为1。
3. 遍历完成后,输出字典中每个字符及其出现次数。
下面是代码示例:
```python
s = "hello world"
d = {}
for c in s:
if c in d:
d[c] += 1
else:
d[c] = 1
for k, v in d.items():
print(k, v)
```
输出结果为:
```
h 1
e 1
l 3
o 2
1
w 1
r 1
d 1
```
相关问题
统计一个数组中所有元素组成的字符串中每个字符出现的次数?
好的,那么可以使用一个哈希表来统计每个字符出现的次数。具体的实现步骤如下:
1. 将数组中的所有元素拼接成一个字符串。
2. 遍历字符串中的每个字符,将字符作为键,在哈希表中查找对应的值,如果存在则将其值加 1,否则添加一个新的键值对,初始值为 1。
3. 遍历完所有字符后,哈希表中存储的就是每个字符出现的次数。
以下是 Python 代码示例:
```python
from collections import defaultdict
def count_chars(arr):
# 将数组中的所有元素拼接成一个字符串
s = ''.join(arr)
# 创建一个默认值为 0 的哈希表
count = defaultdict(int)
# 遍历字符串中的每个字符
for c in s:
# 将字符作为键,在哈希表中查找对应的值
# 如果存在则将其值加 1,否则添加一个新的键值对,初始值为 1
count[c] += 1
# 返回哈希表
return count
```
调用示例:
```python
arr = ['hello', 'world']
result = count_chars(arr)
print(result)
# 输出:defaultdict(<class 'int'>, {'h': 1, 'e': 1, 'l': 3, 'o': 2, 'w': 1, 'r': 1, 'd': 1})
```
其中,`defaultdict(int)` 表示创建一个默认值为 0 的哈希表。
python判断数组中每个字符串结尾
要判断数组中每个字符串结尾,可以使用Python中的字符串方法`endswith()`。该方法接受一个字符串作为参数,判断当前字符串是否以该参数指定的字符串结尾。可以遍历数组中的每个字符串,然后依次调用`endswith()`方法进行判断。以下是示例代码:
```python
arr = ['hello world', 'python', 'apple', 'banana']
suffix = 'ld'
for s in arr:
if s.endswith(suffix):
print(s + ' ends with ' + suffix)
else:
print(s + ' does not end with ' + suffix)
```
输出结果为:
```
hello world ends with ld
python does not end with ld
apple does not end with ld
banana ends with ld
```
相关推荐
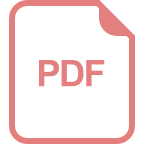
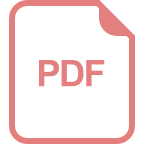
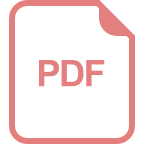












