用c程序给出“链式线性表”的创建 Create、显示 Display、插 入 Insert、删除 Delete、合并 Merge 接口函数
时间: 2024-02-01 17:03:54 浏览: 20
下面是链式线性表的创建、显示、插入、删除和合并函数的示例实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data;
struct ListNode *next;
} ListNode;
// 创建链表
ListNode* createList() {
ListNode *head = NULL, *tail = NULL;
int data;
while (scanf("%d", &data) != EOF) {
ListNode *node = (ListNode*)malloc(sizeof(ListNode));
node->data = data;
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
// 显示链表
void displayList(ListNode *head) {
ListNode *p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 在指定位置插入节点
void insertNode(ListNode *head, int index, int data) {
ListNode *p = head, *prev = NULL;
int i = 0;
while (p != NULL && i < index) {
prev = p;
p = p->next;
i++;
}
if (p == NULL && i < index) {
printf("插入位置超出链表长度\n");
return;
}
ListNode *node = (ListNode*)malloc(sizeof(ListNode));
node->data = data;
node->next = p;
if (prev == NULL) {
head = node;
} else {
prev->next = node;
}
}
// 删除指定位置的节点
void deleteNode(ListNode *head, int index) {
ListNode *p = head, *prev = NULL;
int i = 0;
while (p != NULL && i < index) {
prev = p;
p = p->next;
i++;
}
if (p == NULL && i < index) {
printf("删除位置超出链表长度\n");
return;
}
if (prev == NULL) {
head = p->next;
} else {
prev->next = p->next;
}
free(p);
}
// 合并两个链表
ListNode* mergeList(ListNode *head1, ListNode *head2) {
ListNode *p1 = head1, *p2 = head2, *head = NULL, *tail = NULL;
while (p1 != NULL && p2 != NULL) {
ListNode *node = (ListNode*)malloc(sizeof(ListNode));
if (p1->data < p2->data) {
node->data = p1->data;
p1 = p1->next;
} else {
node->data = p2->data;
p2 = p2->next;
}
node->next = NULL;
if (head == NULL) {
head = node;
tail = node;
} else {
tail->next = node;
tail = node;
}
}
if (p1 != NULL) {
tail->next = p1;
}
if (p2 != NULL) {
tail->next = p2;
}
return head;
}
// 测试函数
int main() {
// 创建链表
printf("请输入链表1的元素,以-1结束:\n");
ListNode *list1 = createList();
printf("链表1为:\n");
displayList(list1);
printf("请输入链表2的元素,以-1结束:\n");
ListNode *list2 = createList();
printf("链表2为:\n");
displayList(list2);
// 插入节点
printf("在链表1的第2个位置插入元素4:\n");
insertNode(list1, 2, 4);
displayList(list1);
// 删除节点
printf("删除链表1的第3个元素:\n");
deleteNode(list1, 3);
displayList(list1);
// 合并链表
printf("合并链表1和链表2:\n");
ListNode *list3 = mergeList(list1, list2);
printf("合并后的链表为:\n");
displayList(list3);
return 0;
}
```
注意,这只是一个示例实现,实际使用时需要根据具体需求进行修改。
相关推荐
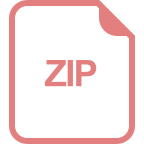








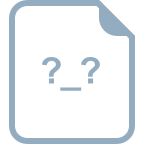
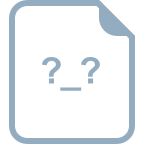
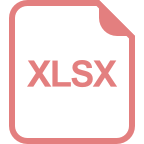