python selenium 多次执行
时间: 2023-07-12 20:57:23 浏览: 210
如果你想在 Python Selenium 中多次执行某个操作,可以使用循环语句。例如,如果你想在一个页面中点击多次某个按钮,可以使用 for 循环语句来实现:
```python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
# 打开浏览器
driver = webdriver.Chrome()
# 打开页面
driver.get("http://www.example.com")
# 找到按钮元素
button = driver.find_element_by_xpath("//button[@id='my-button']")
# 点击按钮 5 次
for i in range(5):
button.click()
# 关闭浏览器
driver.close()
```
在上面的代码中,我们使用了 for 循环语句,通过点击按钮 5 次来演示了多次执行操作的方法。你可以使用类似的方法来实现你的多次操作需求。
相关问题
python selenium滑动页面
使用Selenium可以通过模拟鼠标操作来滑动页面。具体步骤如下:
1. 导入Selenium库和ActionChains类:
```python
from selenium import webdriver
from selenium.webdriver.common.action_chains import ActionChains
```
2. 创建一个WebDriver对象:
```python
driver = webdriver.Chrome()
```
3. 打开需要滑动的页面:
```python
driver.get('https://www.example.com')
```
4. 找到需要滑动的元素:
```python
element = driver.find_element_by_xpath('//div[@class="scrollable"]')
```
5. 创建一个ActionChains对象:
```python
actions = ActionChains(driver)
```
6. 使用ActionChains对象进行滑动操作:
```python
actions.move_to_element(element).click_and_hold().move_by_offset(0, 100).release().perform()
```
其中,move_to_element()方法将鼠标移动到指定元素上,click_and_hold()方法按下鼠标左键,move_by_offset()方法移动鼠标到指定位置,release()方法释放鼠标左键,perform()方法执行操作。
7. 可以多次执行上述操作来实现连续滑动。
```python
actions.move_to_element(element).click_and_hold().move_by_offset(0, 100).release().perform()
actions.move_to_element(element).click_and_hold().move_by_offset(0, 100).release().perform()
```
8. 最后关闭WebDriver对象:
```python
driver.quit()
```
阅读全文
相关推荐






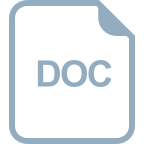


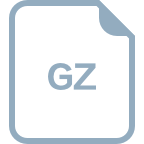



