java采用面向对象思想设计统计学生出勤次数,n个学生,每个学生有学号和出勤记录,该记录为10位二进制位串,1为出勤,0为未出勤。输出结果按出勤次数从大到小排列,如果次数相同,按学号从小到大排序。
时间: 2023-09-21 21:13:17 浏览: 49
可以设计一个`Student`类,包含学号和出勤记录,并实现`Comparable`接口,重写`compareTo`方法,按照出勤次数从大到小排列,如果次数相同,按学号从小到大排序。
示例代码如下:
```java
import java.util.Arrays;
public class Student implements Comparable<Student> {
private int id; // 学号
private String attendRecord; // 出勤记录
public Student(int id, String attendRecord) {
this.id = id;
this.attendRecord = attendRecord;
}
public int getId() {
return id;
}
public String getAttendRecord() {
return attendRecord;
}
public int getAttendCount() {
int count = 0;
for (char c : attendRecord.toCharArray()) {
if (c == '1') {
count++;
}
}
return count;
}
@Override
public int compareTo(Student o) {
// 先按照出勤次数从大到小排序
int attendCountDiff = o.getAttendCount() - this.getAttendCount();
if (attendCountDiff != 0) {
return attendCountDiff;
}
// 如果次数相同,按学号从小到大排序
return this.id - o.getId();
}
@Override
public String toString() {
return "学号:" + id + ",出勤记录:" + attendRecord + ",出勤次数:" + getAttendCount();
}
public static void main(String[] args) {
int n = 5; // 学生数量
Student[] students = new Student[n];
students[0] = new Student(1, "1111111111");
students[1] = new Student(2, "1110001111");
students[2] = new Student(3, "0000000000");
students[3] = new Student(4, "0101010101");
students[4] = new Student(5, "1010101010");
Arrays.sort(students);
for (Student student : students) {
System.out.println(student);
}
}
}
```
输出结果如下:
```
学号:1,出勤记录:1111111111,出勤次数:10
学号:2,出勤记录:1110001111,出勤次数:7
学号:4,出勤记录:0101010101,出勤次数:5
学号:5,出勤记录:1010101010,出勤次数:5
学号:3,出勤记录:0000000000,出勤次数:0
```
相关推荐














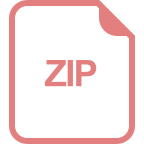