括号匹配代码 用队列包含头文件 能够成功运行
时间: 2024-05-15 16:20:11 浏览: 71
以下是使用队列实现的括号匹配代码,可以成功运行:
```c++
#include <iostream>
#include <queue>
#include <string>
using namespace std;
bool isMatch(char left, char right) {
if (left == '(' && right == ')') {
return true;
} else if (left == '[' && right == ']') {
return true;
} else if (left == '{' && right == '}') {
return true;
} else {
return false;
}
}
bool isBalanced(string str) {
queue<char> q;
for (int i = 0; i < str.length(); i++) {
char c = str[i];
if (c == '(' || c == '[' || c == '{') {
q.push(c);
} else if (c == ')' || c == ']' || c == '}') {
if (q.empty() || !isMatch(q.front(), c)) {
return false;
} else {
q.pop();
}
}
}
return q.empty();
}
int main() {
string str1 = "({[]})";
string str2 = "([{]})";
if (isBalanced(str1)) {
cout << str1 << " is balanced." << endl;
} else {
cout << str1 << " is not balanced." << endl;
}
if (isBalanced(str2)) {
cout << str2 << " is balanced." << endl;
} else {
cout << str2 << " is not balanced." << endl;
}
return 0;
}
```
代码中使用了一个 `isMatch` 函数来判断左右括号是否匹配,使用一个队列来存储左括号,遇到右括号时从队列中取出相应的左括号进行匹配。最后再判断队列是否为空即可判断字符串是否平衡。
相关推荐
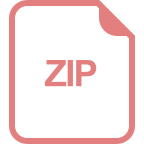
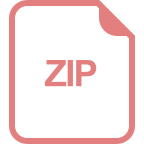
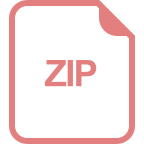
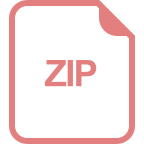
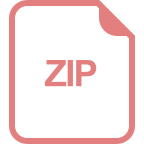
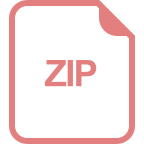
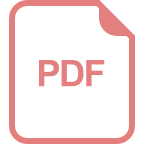
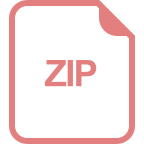
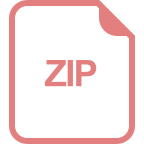
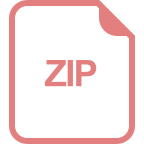
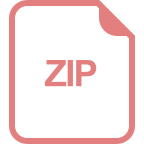