skimage.segmentation 的mark_boundaries怎么用
时间: 2023-10-01 20:03:16 浏览: 939
`mark_boundaries` 是 `skimage.segmentation` 中的一个函数,用于在图像的边界处绘制轮廓线。它可以接受两个参数,第一个参数是原始图像,第二个参数是对应的标签图像,它们的形状应该相同。标签图像中每个像素的值表示原始图像中对应像素所属的区域编号。
下面是一个使用 `mark_boundaries` 函数的例子:
```python
from skimage.segmentation import mark_boundaries
from skimage.io import imread
# 读入一张图像
img = imread('image.jpg')
# 对图像进行分割并得到标签图像
labels = segmentation.slic(img, n_segments=100, compactness=10)
# 在图像边界处绘制轮廓线
boundaries = mark_boundaries(img, labels, color=(1, 0, 0))
# 将结果显示出来
plt.imshow(boundaries)
plt.show()
```
在这个例子中,我们首先读入一张图像,并使用 SLIC 算法对其进行分割,得到标签图像。然后,使用 `mark_boundaries` 函数在图像边界处绘制轮廓线,并将结果显示出来。
需要注意的是,`mark_boundaries` 函数默认使用黑色绘制轮廓线,如果需要使用其他颜色,可以通过 `color` 参数指定。在这个例子中,我们将轮廓线的颜色设置为红色。
相关问题
from skimage.segmentation import slic from skimage.segmentation import mark_boundaries from skimage.util import img_as_float import matplotlib.pyplot as plt import numpy as np import cv2 # args args = {"image": 'I:\\18Breakageratecalculation\\SVM run\\images\\001.jpg'} # load the image and apply SLIC and extract (approximately) # the supplied number of segments image = cv2.imread(args["image"]) segments = slic(img_as_float(image), n_segments=100, sigma=5) # show the output of SLIC fig = plt.figure('Superpixels') ax = fig.add_subplot(1, 1, 1) ax.imshow(mark_boundaries(img_as_float(cv2.cvtColor(image, cv2.COLOR_BGR2RGB)), segments)) plt.axis("off") plt.show() print("segments:\n", segments) print("np.unique(segments):", np.unique(segments)) # loop over the unique segment values for (i, segVal) in enumerate(np.unique(segments)): # construct a mask for the segment print("[x] inspecting segment {}, for {}".format(i, segVal)) mask = np.zeros(image.shape[:2], dtype="uint8") mask[segments == segVal] = 255 # show the masked region cv2.imshow("Mask", mask) cv2.imshow("Applied", np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0)) cv2.waitKey(0)怎么保存超像素块
要保存超像素块,你可以在循环中将每个超像素块保存为一个单独的图像文件。例如,你可以在循环内部使用以下代码将每个超像素块保存为一个单独的文件:
```python
cv2.imwrite("segment_%d.png" % i, np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0))
```
这将使用当前循环索引 i 作为文件名的一部分,将每个超像素块保存为一个名为 "segment_i.png" 的文件。
有两张大小相同的图像A和B,利用代码:from skimage.segmentation import slic from skimage.segmentation import mark_boundaries from skimage.util import img_as_float import matplotlib.pyplot as plt import numpy as np import cv2 # args args = {"image": './1.png'} # load the image and apply SLIC and extract (approximately) # the supplied number of segments image = cv2.imread(args["image"]) segments = slic(img_as_float(image), n_segments=100, sigma=5) # show the output of SLIC fig = plt.figure('Superpixels') ax = fig.add_subplot(1, 1, 1) ax.imshow(mark_boundaries(img_as_float(cv2.cvtColor(image, cv2.COLOR_BGR2RGB)), segments)) plt.axis("off") plt.show() print("segments:\n", segments) print("np.unique(segments):", np.unique(segments)) # loop over the unique segment values for (i, segVal) in enumerate(np.unique(segments)): # construct a mask for the segment print("[x] inspecting segment {}, for {}".format(i, segVal)) mask = np.zeros(image.shape[:2], dtype="uint8") mask[segments == segVal] = 255 print(mask.shape) # show the masked region cv2.imshow("Mask", mask) cv2.imshow("Applied", np.multiply(image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0)) cv2.waitKey(0),对A进行超像素分割,将A划分的每个超像素块范围进行记录,应用到B上,使B直接得到超像素图像。给出pytorch实现代码
以下是使用PyTorch实现将图像B应用超像素图像A的代码:
```python
import torch
import numpy as np
import cv2
import matplotlib.pyplot as plt
from skimage.segmentation import slic, mark_boundaries
from skimage.util import img_as_float
# Load the images
image_a_path = './image_a.png'
image_b_path = './image_b.png'
image_a = cv2.imread(image_a_path)
image_b = cv2.imread(image_b_path)
# Generate superpixels for image A
segments_a = slic(img_as_float(image_a), n_segments=100, sigma=5)
# Loop over the unique segment values for image A
for seg_val in np.unique(segments_a):
# Construct a mask for the segment
mask = np.zeros(image_a.shape[:2], dtype="uint8")
mask[segments_a == seg_val] = 255
# Apply the mask to image B
masked_image_b = np.multiply(image_b, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0)
# Convert the masked image to a PyTorch tensor
tensor_image = torch.from_numpy(masked_image_b.astype(np.float32)).permute(2, 0, 1) / 255.0
# TODO: Apply your PyTorch model to the tensor_image
# Convert the output tensor to a masked image
output_image = tensor_image.permute(1, 2, 0).numpy() * 255.0
output_image = output_image.astype(np.uint8)
output_image = np.multiply(output_image, cv2.cvtColor(mask, cv2.COLOR_GRAY2BGR) > 0)
# Show the output image
fig = plt.figure('Output')
ax = fig.add_subplot(1, 1, 1)
ax.imshow(output_image)
plt.axis("off")
plt.show()
```
在上面的代码中,我们首先加载了图像A和图像B。然后,我们使用skimage.segmentation中的slic函数生成图像A的超像素图像。对于每个超像素块,我们构造一个掩膜并将其应用到图像B上。然后,我们将掩膜后的图像转换为PyTorch张量,并将其输入到您的PyTorch模型中。最后,我们将输出张量转换回图像格式,并将其与掩膜相乘以获得最终输出图像。
阅读全文
相关推荐
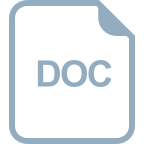
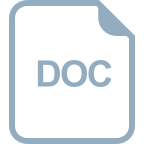
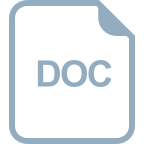






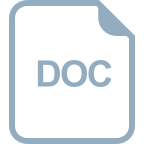
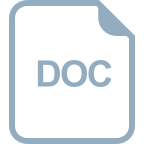
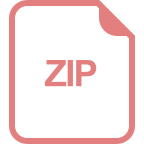
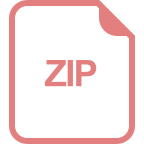
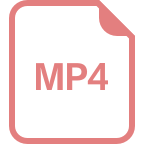