以下是驱动开发相关问题:给定一个文件,在文件其中包含数字,英文字符串和中文三种数据,需要对此文件进行数据提取并格式化输出,请用C++实现
时间: 2024-02-20 08:57:15 浏览: 21
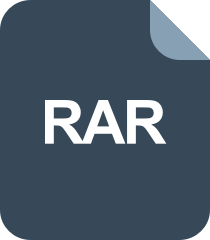
基于C++的文件读取排版(包含字符和数字)

可以使用C++的文件输入输出流来读取和写入文件,同时使用正则表达式来匹配数字、英文字符串和中文。
以下是一个简单的实现示例:
```c++
#include <iostream>
#include <fstream>
#include <regex>
#include <string>
using namespace std;
int main() {
ifstream infile("input.txt");
ofstream outfile("output.txt");
if (!infile) {
cout << "Error opening input file" << endl;
return 1;
}
if (!outfile) {
cout << "Error opening output file" << endl;
return 1;
}
// 正则表达式匹配数字、英文字符串和中文
regex num_pattern("\\d+");
regex eng_pattern("[A-Za-z]+");
regex chi_pattern("[\\u4e00-\\u9fa5]+");
string line;
while (getline(infile, line)) {
// 匹配数字
smatch num_match;
if (regex_search(line, num_match, num_pattern)) {
// 输出匹配到的数字
for (auto x : num_match) {
outfile << x << " ";
}
}
// 匹配英文字符串
smatch eng_match;
if (regex_search(line, eng_match, eng_pattern)) {
// 输出匹配到的英文字符串
for (auto x : eng_match) {
outfile << x << " ";
}
}
// 匹配中文
smatch chi_match;
if (regex_search(line, chi_match, chi_pattern)) {
// 输出匹配到的中文
for (auto x : chi_match) {
outfile << x << " ";
}
}
outfile << endl;
}
infile.close();
outfile.close();
return 0;
}
```
这个程序会读取名为 "input.txt" 的文件,并将匹配到的数字、英文字符串和中文格式化输出到名为 "output.txt" 的文件中。
阅读全文
相关推荐
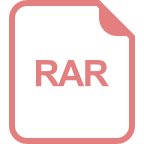
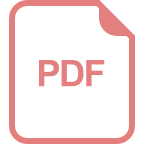
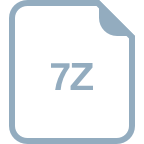
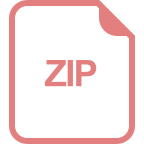
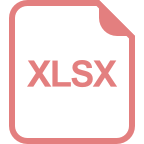
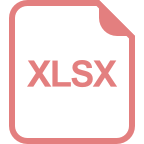
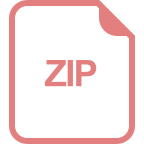
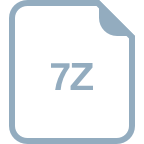
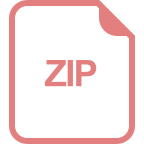
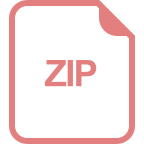
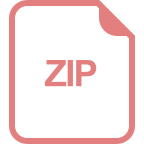
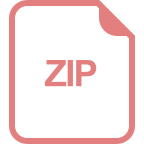