利用Java语言编写不定长子网划分程序,输入需要划分的多个子网,并列出所有可用子网以及各子网IP范围
时间: 2024-05-09 11:15:49 浏览: 44
以下是一个简单的Java程序,可以实现不定长子网划分,并列出各子网IP范围:
```java
import java.util.Scanner;
public class SubnetCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入 IP 地址(例:192.168.0.1):");
String[] ipAddress = scanner.nextLine().split("\\.");
int[] binaryIP = new int[32];
for (int i = 0; i < 4; i++) {
int octet = Integer.parseInt(ipAddress[i]);
for (int j = 7; j >= 0; j--) {
binaryIP[8 * i + 7 - j] = (octet >> j) & 1;
}
}
System.out.print("请输入子网掩码(例:255.255.255.0):");
String[] subnetMask = scanner.nextLine().split("\\.");
int[] binaryMask = new int[32];
for (int i = 0; i < 4; i++) {
int octet = Integer.parseInt(subnetMask[i]);
for (int j = 7; j >= 0; j--) {
binaryMask[8 * i + 7 - j] = (octet >> j) & 1;
}
}
int subnetBits = 0;
for (int i = 0; i < 32; i++) {
if (binaryMask[i] == 1) {
subnetBits++;
} else {
break;
}
}
int numSubnets = (int) Math.pow(2, subnetBits);
int numHosts = (int) Math.pow(2, 32 - subnetBits) - 2;
System.out.println("可用子网数:" + numSubnets);
System.out.println("每个子网可用主机数:" + numHosts);
System.out.print("请输入需要划分的子网数:");
int numDesiredSubnets = scanner.nextInt();
int bitsToBorrow = (int) Math.ceil(Math.log(numDesiredSubnets) / Math.log(2));
int newSubnetBits = subnetBits + bitsToBorrow;
int newNumSubnets = (int) Math.pow(2, newSubnetBits);
int newNumHosts = (int) Math.pow(2, 32 - newSubnetBits) - 2;
System.out.println("需要借用 " + bitsToBorrow + " 位来划分 " + numDesiredSubnets + " 个子网");
System.out.println("新子网掩码为:" + binaryMaskString(newSubnetBits));
System.out.println("每个新子网可用主机数:" + newNumHosts);
int[][] subnets = new int[numDesiredSubnets][32];
int[][] ranges = new int[numDesiredSubnets][2];
for (int i = 0; i < numDesiredSubnets; i++) {
for (int j = 0; j < 32; j++) {
if (j < subnetBits) {
subnets[i][j] = binaryIP[j];
} else if (j < newSubnetBits) {
subnets[i][j] = 0;
} else {
subnets[i][j] = (i >> (j - newSubnetBits)) & 1;
}
}
ranges[i][0] = binaryIPToInt(subnets[i]);
ranges[i][1] = ranges[i][0] + newNumHosts + 1;
}
System.out.println("子网\t起始地址\t结束地址");
for (int i = 0; i < numDesiredSubnets; i++) {
System.out.println((i + 1) + "\t" + intToIPAddress(ranges[i][0]) + "\t" + intToIPAddress(ranges[i][1] - 1));
}
}
private static String binaryMaskString(int bits) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 32; i++) {
if (i < bits) {
sb.append("1");
} else {
sb.append("0");
}
if (i % 8 == 7) {
sb.append(".");
}
}
return sb.toString().substring(0, sb.length() - 1);
}
private static int binaryIPToInt(int[] binaryIP) {
int num = 0;
for (int i = 0; i < 32; i++) {
num += binaryIP[i] * Math.pow(2, 31 - i);
}
return num;
}
private static String intToIPAddress(int num) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 4; i++) {
sb.append(num / (int) Math.pow(256, 3 - i)).append(".");
num %= (int) Math.pow(256, 3 - i);
}
return sb.toString().substring(0, sb.length() - 1);
}
}
```
运行程序,按照提示输入 IP 地址和子网掩码,然后输入需要划分的子网数。程序会输出可用子网数、每个子网可用主机数、需要借用的位数、新子网掩码、每个新子网可用主机数以及各子网的起始地址和结束地址。
阅读全文
相关推荐



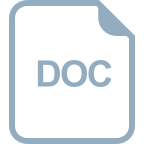
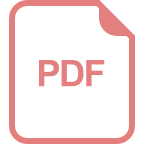









