输入一行句子,数出句子中有多少个单词并输出,再让用户输入一串数字表示单词重新排序的顺序,最后按用户设定的顺序来输出一行新的句子c++
时间: 2024-11-30 07:20:58 浏览: 5
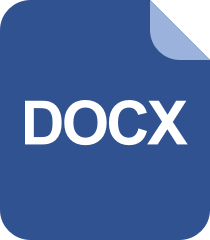
单词排序(计蒜客)
在C++中,你可以通过标准库`std::istringstream`和`std::string`来实现这个功能。首先,你需要读取句子,计算单词数量,然后接受用户输入的排序序列。下面是一个简单的示例:
```cpp
#include <iostream>
#include <sstream>
#include <vector>
#include <algorithm>
int count_words(const std::string& sentence) {
std::istringstream iss(sentence);
std::string word;
int count = 0;
while (iss >> word) {
count++;
}
return count;
}
void sort_sentence(std::vector<int>& order, std::string& sentence) {
std::vector<std::pair<int, std::string>> words(order.size());
for (size_t i = 0; i < order.size(); i++) {
std::getline(sentence, words[i].second, ' ');
words[i].first = order[i];
}
std::sort(words.begin(), words.end(),
[](const std::pair<int, std::string>& a, const std::pair<int, std::string>& b) {
return a.first < b.first;
});
sentence.clear();
for (const auto& pair : words) {
sentence += pair.second + " ";
}
sentence.pop_back(); // 移除最后一个多余的空格
}
int main() {
std::string input_sentence;
std::cout << "请输入一个句子: ";
std::getline(std::cin, input_sentence);
int word_count = count_words(input_sentence);
std::cout << "句子中有 " << word_count << " 个单词。\n";
std::vector<int> order;
std::cout << "请输入单词重新排序的序号(以空格分隔): ";
std::string input_order;
std::getline(std::cin, input_order);
std::istringstream isort(input_order);
for (int i; isort >> i; ) {
order.push_back(i);
}
sort_sentence(order, input_sentence);
std::cout << "按照新顺序的句子是: " << input_sentence << "\n";
return 0;
}
```
在这个程序中,我们首先读取句子,并计算单词数量。接着,用户输入排序顺序,我们将这些顺序与原始单词对应起来。然后对这些单词按照用户提供的顺序进行排序,并输出结果。
阅读全文
相关推荐
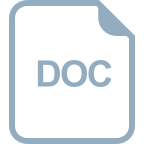
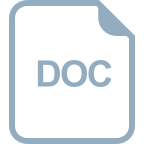






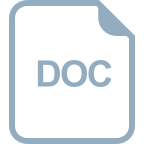
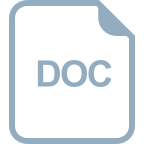