react子路由动态渲染
时间: 2023-09-08 22:13:40 浏览: 83
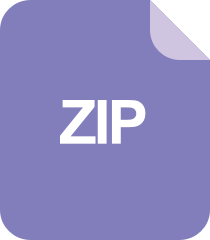
react-dynamic-import:使用动态导入动态加载和渲染任何React模块(组件或HOC)

在React中,可以使用React Router来实现路由功能。对于子路由的动态渲染,可以通过以下步骤实现:
1. 在父组件中定义子路由的路径和组件。
```JavaScript
import { Switch, Route } from 'react-router-dom';
const ParentComponent = () => (
<div>
{/* 父组件内容 */}
<Switch>
<Route path="/parent/child1" component={Child1Component} />
<Route path="/parent/child2/:id" component={Child2Component} />
</Switch>
</div>
);
```
2. 在子组件中获取父组件传递的参数。
```JavaScript
const Child2Component = ({ match }) => {
const { id } = match.params;
return (
<div>
{/* 子组件内容 */}
<p>子路由参数:{id}</p>
</div>
);
};
```
3. 在父组件中使用Link组件来跳转到子路由。
```JavaScript
import { Link } from 'react-router-dom';
const ParentComponent = () => (
<div>
{/* 父组件内容 */}
<ul>
<li><Link to="/parent/child1">子路由1</Link></li>
<li><Link to="/parent/child2/123">子路由2</Link></li>
</ul>
<Switch>
<Route path="/parent/child1" component={Child1Component} />
<Route path="/parent/child2/:id" component={Child2Component} />
</Switch>
</div>
);
```
4. 在子组件中使用Link组件来返回父组件。
```JavaScript
import { Link } from 'react-router-dom';
const Child2Component = ({ match }) => {
const { id } = match.params;
return (
<div>
{/* 子组件内容 */}
<Link to="/parent">返回父路由</Link>
<p>子路由参数:{id}</p>
</div>
);
};
```
以上就是React子路由动态渲染的实现步骤。
阅读全文
相关推荐
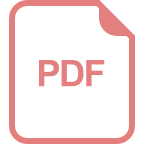
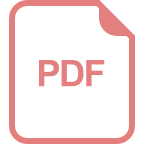
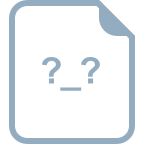
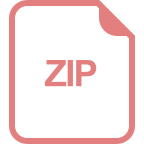
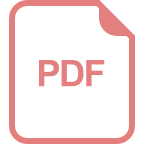
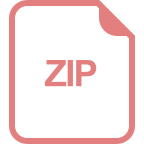
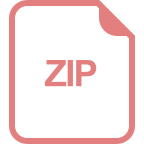
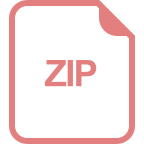
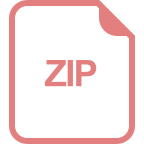
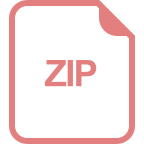
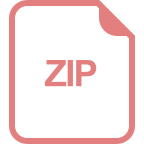
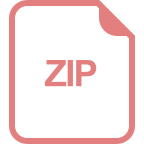
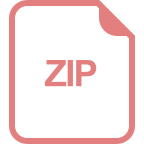
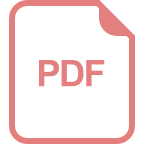
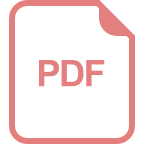
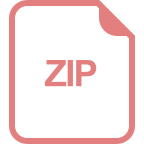
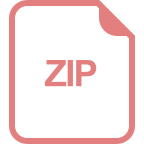