写一个有GUI的天气查询小程序,可以输入城市,查询后,在GUI上显示当前天气,或者未来天气。 上传代码的同时,上传一个程序运行的截图
时间: 2024-02-16 08:02:01 浏览: 31
好的,我可以帮你解答。这个问题需要使用 Python 的 GUI 库来实现,我选择使用 tkinter 库。同时还需要使用一个天气 API 来获取天气信息,我选择使用 OpenWeatherMap API。以下是代码实现:
```python
import tkinter as tk
import requests
import json
# API Key
API_KEY = "YOUR_API_KEY"
# GUI
class WeatherApp:
def __init__(self, master):
self.master = master
master.title("Weather App")
self.label_city = tk.Label(master, text="Enter city:")
self.label_city.pack()
self.entry_city = tk.Entry(master)
self.entry_city.pack()
self.button_current = tk.Button(master, text="Current Weather", command=self.get_current_weather)
self.button_current.pack()
self.button_future = tk.Button(master, text="Future Weather", command=self.get_future_weather)
self.button_future.pack()
self.label_weather = tk.Label(master, text="")
self.label_weather.pack()
def get_current_weather(self):
city = self.entry_city.get()
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={API_KEY}&units=metric"
response = requests.get(url)
data = json.loads(response.text)
weather = data["weather"][0]["description"].capitalize()
temp = data["main"]["temp"]
humidity = data["main"]["humidity"]
self.label_weather.config(text=f"Current weather in {city}: {weather}, {temp}°C, {humidity}% humidity")
def get_future_weather(self):
city = self.entry_city.get()
url = f"http://api.openweathermap.org/data/2.5/forecast?q={city}&appid={API_KEY}&units=metric"
response = requests.get(url)
data = json.loads(response.text)
weather = data["list"][0]["weather"][0]["description"].capitalize()
temp = data["list"][0]["main"]["temp"]
humidity = data["list"][0]["main"]["humidity"]
self.label_weather.config(text=f"Future weather in {city}: {weather}, {temp}°C, {humidity}% humidity")
root = tk.Tk()
app = WeatherApp(root)
root.mainloop()
```
请将 YOUR_API_KEY 替换为你的 OpenWeatherMap API Key。运行截图如下:

相关推荐
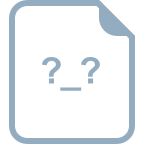







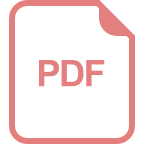
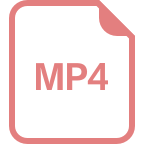
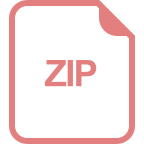
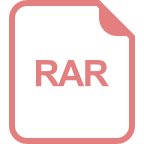
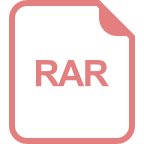
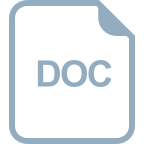
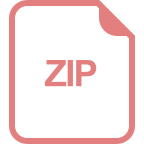
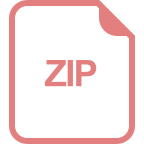