仓库管理编写一个仓库管理程序管理仓库内货物的信息。仓库中货物信息的组成部分为:货物编号、货物名称、货物单价、货物数量等。1)增加或删除货物信息2)按编号或名称查询货物信息3)显示库存量不足一定值(由用房从键盘输入)的货物信息4)显示所有货物的信息5)用子函数实现各个子功能输入:程序正常运行后,屏幕上显示一个文字菜单(根据序号选定相应的操作项目),当用房选定操作项目所对应的序号时,根据应用程序的提示信息,从键盘上输入相应的数据。输出:1)应用程序正常运行后,要在屏幕上显示一个文字菜单2)要求用房输入数据时,要给出清晰、明确的提示信息,包括输入的数据内容、格式及结束方式等。
时间: 2024-03-13 13:45:42 浏览: 12
好的,这是一个比较简单的仓库管理程序,您可以参考以下代码实现:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// 定义货物类
class Goods {
public:
Goods(int id, string name, double price, int quantity)
: id(id), name(name), price(price), quantity(quantity) {}
int get_id() const { return id; }
string get_name() const { return name; }
double get_price() const { return price; }
int get_quantity() const { return quantity; }
void add_quantity(int amount) { quantity += amount; }
void subtract_quantity(int amount) { quantity -= amount; }
private:
int id;
string name;
double price;
int quantity;
};
// 定义仓库类
class Warehouse {
public:
// 增加货物
void add_goods(int id, string name, double price, int quantity) {
goods_list.emplace_back(id, name, price, quantity);
}
// 删除货物
void delete_goods(int id) {
for (auto it = goods_list.begin(); it != goods_list.end(); ++it) {
if (it->get_id() == id) {
goods_list.erase(it);
break;
}
}
}
// 按编号查询货物
void query_goods_by_id(int id) const {
for (auto it = goods_list.begin(); it != goods_list.end(); ++it) {
if (it->get_id() == id) {
cout << "编号:" << it->get_id() << endl;
cout << "名称:" << it->get_name() << endl;
cout << "单价:" << it->get_price() << endl;
cout << "数量:" << it->get_quantity() << endl;
return;
}
}
cout << "没有找到编号为" << id << "的货物" << endl;
}
// 按名称查询货物
void query_goods_by_name(const string& name) const {
for (auto it = goods_list.begin(); it != goods_list.end(); ++it) {
if (it->get_name() == name) {
cout << "编号:" << it->get_id() << endl;
cout << "名称:" << it->get_name() << endl;
cout << "单价:" << it->get_price() << endl;
cout << "数量:" << it->get_quantity() << endl;
return;
}
}
cout << "没有找到名称为" << name << "的货物" << endl;
}
// 显示库存不足的货物
void show_shortage_goods(int threshold) const {
for (auto it = goods_list.begin(); it != goods_list.end(); ++it) {
if (it->get_quantity() < threshold) {
cout << "编号:" << it->get_id() << endl;
cout << "名称:" << it->get_name() << endl;
cout << "单价:" << it->get_price() << endl;
cout << "数量:" << it->get_quantity() << endl;
}
}
}
// 显示所有货物
void show_all_goods() const {
for (auto it = goods_list.begin(); it != goods_list.end(); ++it) {
cout << "编号:" << it->get_id() << endl;
cout << "名称:" << it->get_name() << endl;
cout << "单价:" << it->get_price() << endl;
cout << "数量:" << it->get_quantity() << endl;
}
}
private:
vector<Goods> goods_list;
};
int main() {
Warehouse warehouse;
while (true) {
cout << endl;
cout << "请选择操作:" << endl;
cout << "1. 增加货物" << endl;
cout << "2. 删除货物" << endl;
cout << "3. 按编号查询货物" << endl;
cout << "4. 按名称查询货物" << endl;
cout << "5. 显示库存不足的货物" << endl;
cout << "6. 显示所有货物" << endl;
cout << "7. 退出程序" << endl;
int choice;
cin >> choice;
if (choice == 1) {
int id, quantity;
string name;
double price;
cout << "请输入货物编号:";
cin >> id;
cout << "请输入货物名称:";
cin >> name;
cout << "请输入货物单价:";
cin >> price;
cout << "请输入货物数量:";
cin >> quantity;
warehouse.add_goods(id, name, price, quantity);
cout << "货物添加成功!" << endl;
}
else if (choice == 2) {
int id;
cout << "请输入要删除的货物编号:";
cin >> id;
warehouse.delete_goods(id);
cout << "货物删除成功!" << endl;
}
else if (choice == 3) {
int id;
cout << "请输入要查询的货物编号:";
cin >> id;
warehouse.query_goods_by_id(id);
}
else if (choice == 4) {
string name;
cout << "请输入要查询的货物名称:";
cin >> name;
warehouse.query_goods_by_name(name);
}
else if (choice == 5) {
int threshold;
cout << "请输入库存下限:";
cin >> threshold;
warehouse.show_shortage_goods(threshold);
}
else if (choice == 6) {
warehouse.show_all_goods();
}
else if (choice == 7) {
break;
}
else {
cout << "无效的操作,请重新输入!" << endl;
}
}
return 0;
}
```
这个程序中,我们定义了一个`Goods`类来存储每个货物的信息,包括编号、名称、单价、数量等。然后我们定义了一个`Warehouse`类来管理所有的货物,包括增加、删除、查询、显示等操作。在`main`函数中,我们使用一个循环来不断接收用户的输入,并执行相应的操作。
相关推荐
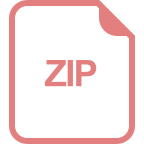
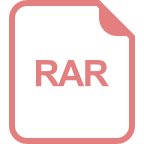


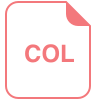
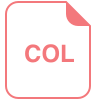
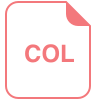










