在python中用遗传算法求解传教士和野人渡河问题,且能够设置野人数量,并且图形化表示
时间: 2024-02-06 15:10:26 浏览: 90
好的,我们可以使用Python中的遗传算法库DEAP(Distributed Evolutionary Algorithms in Python)来实现传教士和野人渡河问题的求解。接下来,我将分步骤介绍如何实现。
1. 安装DEAP库
首先,我们需要在Python环境中安装DEAP库。可以使用以下命令来安装:
```
pip install deap
```
2. 定义问题
在传教士和野人渡河问题中,我们需要将3个传教士和3个野人从一岸运送到另一岸。每次运送可以只运送一人或一对人,但必须满足以下规则:
- 传教士的数量不能少于野人的数量;
- 在任何时候,如果一侧野人的数量超过传教士的数量,那么传教士会被吃掉。
我们可以用一个元组表示每个状态,元组中包括当前岸上的传教士数量、野人数量和船只位置(0表示在左岸,1表示在右岸)。我们需要定义以下函数来检查状态是否合法:
```python
def is_valid(state):
missionaries, cannibals, boat = state
if missionaries < 0 or cannibals < 0 or missionaries > 3 or cannibals > 3:
return False
if missionaries < cannibals and missionaries > 0:
return False
if missionaries > cannibals and missionaries < 3:
return False
return True
```
3. 定义适应度函数
适应度函数用来评价每个个体的适应度,这里我们可以将适应度定义为完成任务所需的步数。我们可以使用BFS算法来搜索从初始状态到目标状态的最短路径,然后将路径长度作为适应度。
```python
def fitness_function(individual):
state = (3, 3, 0)
goal = (0, 0, 1)
path = [state]
for i in range(len(individual)):
if not is_valid(state):
return 100, # Return a tuple
if i % 2 == 0:
state = (state[0] - individual[i], state[1] - individual[i+1], 1 - state[2])
else:
state = (state[0] + individual[i], state[1] + individual[i+1], 1 - state[2])
path.append(state)
if state == goal:
return len(path),
return 100,
```
4. 定义遗传算法参数
接下来,我们需要定义遗传算法的参数。这里我们将使用DEAP库提供的工具箱(toolbox)来创建遗传算法所需的函数和参数。
```python
import random
from deap import base, creator, tools
# Set the number of individuals and generations
POPULATION_SIZE = 50
MAX_GENERATIONS = 100
# Set the number of missionaries and cannibals
NUM_MISSIONARIES = 3
NUM_CANNIBALS = 3
# Create the fitness function and individual class
creator.create("FitnessMin", base.Fitness, weights=(-1.0,))
creator.create("Individual", list, fitness=creator.FitnessMin)
# Create the toolbox
toolbox = base.Toolbox()
# Register the mutation and crossover operators
toolbox.register("select", tools.selTournament, tournsize=3)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutFlipBit, indpb=0.1)
# Register the individual generator
toolbox.register("attr_bool", random.randint, 0, 1)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_bool, n=2*(NUM_MISSIONARIES+NUM_CANNIBALS))
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
```
5. 运行遗传算法
现在我们可以开始运行遗传算法来解决传教士和野人渡河问题了。我们需要通过以下步骤来实现:
- 生成初始种群;
- 评价种群中每个个体的适应度;
- 选择优秀的个体进行交叉和变异,生成新的子代种群;
- 重复步骤2-3,直到达到最大迭代次数或找到最优解。
```python
def main():
# Initialize the population
pop = toolbox.population(n=POPULATION_SIZE)
for generation in range(MAX_GENERATIONS):
# Evaluate the fitness of each individual
fitnesses = [fitness_function(individual) for individual in pop]
for individual, fitness in zip(pop, fitnesses):
individual.fitness.values = fitness
# Select the next generation
offspring = toolbox.select(pop, len(pop))
offspring = [toolbox.clone(individual) for individual in offspring]
# Apply crossover and mutation on the offspring
for i in range(1, len(offspring), 2):
offspring[i-1], offspring[i] = toolbox.mate(offspring[i-1], offspring[i])
del offspring[i-1].fitness.values
del offspring[i].fitness.values
for individual in offspring:
toolbox.mutate(individual)
del individual.fitness.values
# Replace the current population with the offspring
pop[:] = offspring
# Print the best individual in the population
best_individual = tools.selBest(pop, k=1)[0]
print("Generation {}: Best Fitness = {}".format(generation+1, best_individual.fitness.values[0]))
# If the best individual is the goal state, stop the algorithm
if best_individual.fitness.values[0] == 0:
break
print("Best Individual: ", best_individual)
```
6. 可视化结果
最后,我们可以使用matplotlib库来可视化每次迭代的最优适应度值。下面是一个简单的例子:
```python
import matplotlib.pyplot as plt
def plot_fitness_progress(fitness_progress):
plt.plot(fitness_progress)
plt.xlabel("Generation")
plt.ylabel("Fitness")
plt.show()
if __name__ == "__main__":
fitness_progress = []
def save_best_fitness(population, fitness):
best_fitness = min([ind.fitness.values[0] for ind in population])
fitness_progress.append(best_fitness)
# Add the callback function to the toolbox
toolbox.decorate("mate", save_best_fitness)
toolbox.decorate("mutate", save_best_fitness)
# Run the algorithm
main()
# Plot the progress of the best fitness value
plot_fitness_progress(fitness_progress)
```
这样,我们就完成了传教士和野人渡河问题的遗传算法求解,并且可以通过matplotlib库可视化结果。
阅读全文
相关推荐
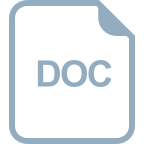
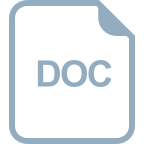
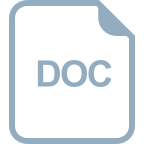
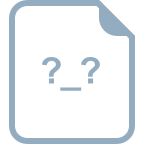
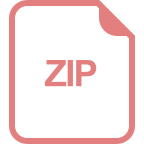
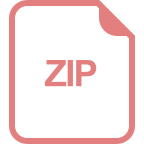
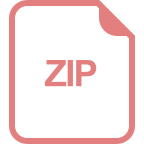
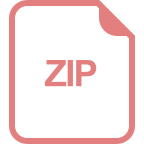
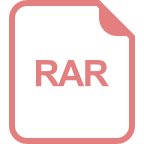
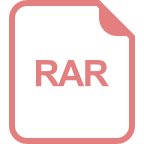
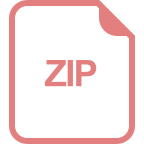
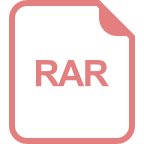
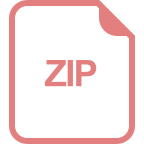
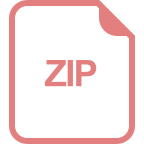
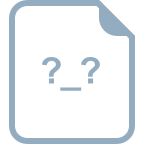