请用C++语言调用cryptto库编写一个字符串AESl加解密的函数
时间: 2023-09-03 13:27:17 浏览: 81
### 回答1:
可以使用以下C语言代码来编写一个字符串AES加解密的函数:#include <stdio.h>
#include <string.h>
#include <openssl/aes.h>
void aes_encrypt(char *plaintext, char *ciphertext)
{
AES_KEY enc_key;
unsigned char key[AES_BLOCK_SIZE];
unsigned char iv[AES_BLOCK_SIZE];
memset(key, 0x00, AES_BLOCK_SIZE);
memset(iv, 0x00, AES_BLOCK_SIZE);
AES_set_encrypt_key(key, AES_BLOCK_SIZE * 8, &enc_key);
AES_cbc_encrypt((unsigned char *)plaintext, (unsigned char *)ciphertext, strlen(plaintext), &enc_key, iv, AES_ENCRYPT);
}
void aes_decrypt(char *ciphertext, char *plaintext)
{
AES_KEY dec_key;
unsigned char key[AES_BLOCK_SIZE];
unsigned char iv[AES_BLOCK_SIZE];
memset(key, 0x00, AES_BLOCK_SIZE);
memset(iv, 0x00, AES_BLOCK_SIZE);
AES_set_decrypt_key(key, AES_BLOCK_SIZE * 8, &dec_key);
AES_cbc_encrypt((unsigned char *)ciphertext, (unsigned char *)plaintext, strlen(ciphertext), &dec_key, iv, AES_DECRYPT);
}
### 回答2:
使用C语言调用crypto库编写一个字符串AES加解密的函数,可以参考以下示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/aes.h>
#define AES_KEY_SIZE 256
#define BLOCK_SIZE 16
// AES加密函数
int aes_encrypt(const unsigned char *plaintext, size_t plaintext_len, const unsigned char *key, unsigned char *ciphertext) {
AES_KEY aes_key;
if (AES_set_encrypt_key(key, AES_KEY_SIZE, &aes_key) < 0) {
return -1; // 密钥设置失败
}
size_t num_blocks = (plaintext_len + BLOCK_SIZE - 1) / BLOCK_SIZE;
unsigned char iv[BLOCK_SIZE];
memset(iv, 0, sizeof(iv));
for (size_t i = 0; i < num_blocks; i++) {
AES_cbc_encrypt(plaintext + i * BLOCK_SIZE, ciphertext + i * BLOCK_SIZE, BLOCK_SIZE, &aes_key, iv, AES_ENCRYPT);
}
return num_blocks * BLOCK_SIZE;
}
// AES解密函数
int aes_decrypt(const unsigned char *ciphertext, size_t ciphertext_len, const unsigned char *key, unsigned char *plaintext) {
AES_KEY aes_key;
if (AES_set_decrypt_key(key, AES_KEY_SIZE, &aes_key) < 0) {
return -1; // 密钥设置失败
}
size_t num_blocks = ciphertext_len / BLOCK_SIZE;
unsigned char iv[BLOCK_SIZE];
memset(iv, 0, sizeof(iv));
for (size_t i = 0; i < num_blocks; i++) {
AES_cbc_encrypt(ciphertext + i * BLOCK_SIZE, plaintext + i * BLOCK_SIZE, BLOCK_SIZE, &aes_key, iv, AES_DECRYPT);
}
return num_blocks * BLOCK_SIZE;
}
int main() {
const unsigned char *plaintext = "Hello, world!";
const unsigned char *key = "0123456789abcdef";
size_t plaintext_len = strlen(plaintext);
// 加密示例
unsigned char ciphertext[plaintext_len];
int encrypted_len = aes_encrypt(plaintext, plaintext_len, key, ciphertext);
printf("Encrypted text: ");
for (int i = 0; i < encrypted_len; ++i) {
printf("%02x", ciphertext[i]);
}
printf("\n");
// 解密示例
unsigned char decrypted[encrypted_len];
int decrypted_len = aes_decrypt(ciphertext, encrypted_len, key, decrypted);
printf("Decrypted text: %s\n", decrypted);
return 0;
}
```
上述代码使用了OpenSSL库提供的AES加密和解密函数,其中密钥长度为256位,加密模式为CBC,填充模式为PKCS#7。在示例中,我们加密了字符串"Hello, world!",并将加密后的结果打印出来,然后再解密得到原始字符串。你可以按照自己的需求修改输入的明文和密钥。
### 回答3:
使用C语言调用crypto库编写一个字符串AES加解密的函数需要以下步骤:
1. 导入所需的头文件:
```c
#include <stdio.h>
#include <string.h>
#include <openssl/aes.h>
```
2. 定义AES加解密函数:
```c
void aes_encrypt(char *plaintext, char *key, char *ciphertext) {
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_encrypt((unsigned char *)plaintext, (unsigned char *)ciphertext, &aes_key);
}
void aes_decrypt(char *ciphertext, char *key, char *plaintext) {
AES_KEY aes_key;
AES_set_decrypt_key(key, 128, &aes_key);
AES_decrypt((unsigned char *)ciphertext, (unsigned char *)plaintext, &aes_key);
}
```
3. 在主函数中调用AES加解密函数:
```c
int main() {
char plaintext[16] = "Hello, World!";
char key[16] = "abcdefghijklmnop";
char ciphertext[16];
char decrypted[16];
aes_encrypt(plaintext, key, ciphertext);
printf("Ciphertext: %s\n", ciphertext);
aes_decrypt(ciphertext, key, decrypted);
printf("Decrypted: %s\n", decrypted);
return 0;
}
```
在这个例子中,使用了一个16字节的明文字符串"Hello, World!"和一个16字节的密钥字符串"abcdefghijklmnop"。首先调用aes_encrypt函数进行加密,然后打印输出密文。接下来调用aes_decrypt函数进行解密,最后打印输出解密后的明文。
需要注意的是,需要提前安装OpenSSL库并在编译时链接该库,例如使用以下命令进行编译:
```
gcc -o aes aes.c -lcrypto
```
相关推荐
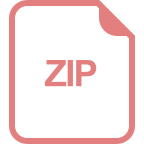


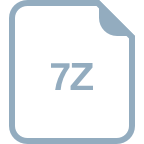
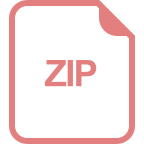
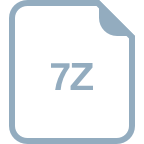
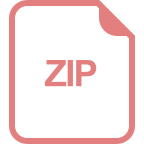
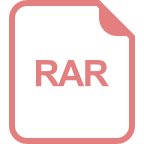
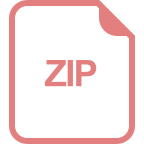
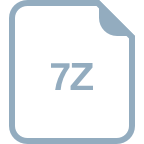
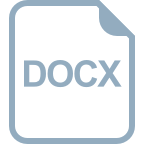
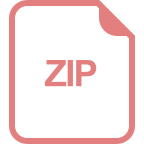
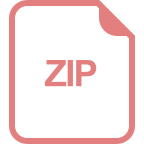
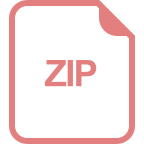
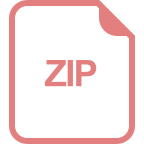
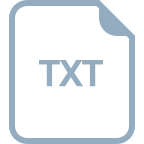
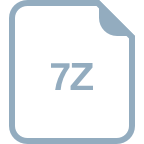