A*算法源码具体解析
时间: 2023-07-10 07:07:35 浏览: 49
A*算法(A-Star算法)是一种常用的寻路算法,它可以在地图中快速找到两个点之间的最短路径。下面是A*算法的具体解析和源码实现。
1.算法原理
A*算法是一种启发式搜索算法,它采用了启发式函数来评估每个搜索状态,从而决定搜索的方向。它的基本思想是在搜索过程中,维护一个开放列表和一个关闭列表,每次选择开放列表中f值最小的状态进行扩展。其中,f值是由g值(起点到当前节点的距离)和h值(当前节点到终点的估价距离)组成的。具体的流程如下:
(1)将起点加入开放列表,初始g值为0,h值为起点到终点的估价距离;
(2)从开放列表中选取f值最小的节点,将其加入关闭列表中;
(3)对该节点的所有相邻节点进行扩展,计算它们的g和h值,将它们加入开放列表中;
(4)如果终点被加入了开放列表,说明搜索结束,返回路径;否则,返回第(2)步。
2.算法优化
A*算法的效率受到启发式函数的影响,如果启发式函数设计不当,可能会导致效率低下,甚至不能找到最优解。为了解决这个问题,可以采用以下优化措施:
(1)使用曼哈顿距离或欧几里得距离作为启发式函数,可以提高搜索效率,同时保证找到最优解;
(2)采用二叉堆等数据结构来实现开放列表,可以提高搜索效率。
3.算法实现
下面是A*算法的Python实现代码:
```python
def astar(start, end, graph):
# 初始化起点和终点
start_node = Node(None, start)
end_node = Node(None, end)
# 初始化开放列表和关闭列表
open_list = [start_node]
closed_list = []
# 循环搜索
while len(open_list) > 0:
# 选取f值最小的节点
current_node = open_list[0]
current_index = 0
for index, item in enumerate(open_list):
if item.f < current_node.f:
current_node = item
current_index = index
# 将当前节点从开放列表中删除,加入关闭列表中
open_list.pop(current_index)
closed_list.append(current_node)
# 如果当前节点是终点,返回路径
if current_node == end_node:
path = []
while current_node is not None:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1]
# 扩展当前节点的相邻节点
neighbors = graph.get_neighbors(current_node.position)
for neighbor in neighbors:
# 如果相邻节点在关闭列表中,跳过
if neighbor in [node.position for node in closed_list]:
continue
# 计算相邻节点的g值和h值
g = current_node.g + graph.get_cost(current_node.position, neighbor)
h = graph.get_cost(neighbor, end_node.position)
f = g + h
# 如果相邻节点不在开放列表中,加入开放列表
neighbor_node = Node(current_node, neighbor)
neighbor_node.g = g
neighbor_node.h = h
neighbor_node.f = f
if neighbor not in [node.position for node in open_list]:
open_list.append(neighbor_node)
# 如果相邻节点已经在开放列表中,更新其g值和f值
else:
for node in open_list:
if node.position == neighbor_node.position:
if node.g > neighbor_node.g:
node.g = neighbor_node.g
node.f = neighbor_node.f
node.parent = current_node
# 如果没有找到路径,返回None
return None
class Node:
def __init__(self, parent=None, position=None):
self.parent = parent
self.position = position
self.g = 0
self.h = 0
self.f = 0
```
其中,graph是一个包含地图信息的类,它具有以下方法:
(1)get_neighbors(position):返回某个位置的相邻位置列表;
(2)get_cost(position1, position2):返回两个位置之间的距离。
4.总结
A*算法是一种有效的寻路算法,它结合了Dijkstra算法和贪心算法的优点,可以在地图中快速找到两个点之间的最短路径。在实现过程中,需要注意启发式函数的设计和开放列表的维护。
相关推荐
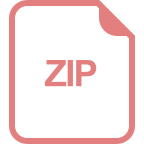
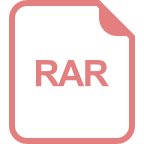














