C语言多线程计算30000000-30001000之间的质数
时间: 2023-07-25 17:41:40 浏览: 201
以下是使用C语言的多线程计算30000000-30001000之间的质数的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 4
#define RANGE_START 30000000
#define RANGE_END 30001000
// 定义线程参数结构体
typedef struct {
int start;
int end;
int *count;
} thread_args;
// 判断一个数是否为质数
int is_prime(int num) {
int i;
if (num <= 1) {
return 0;
}
for (i = 2; i*i <= num; i++) {
if (num % i == 0) {
return 0;
}
}
return 1;
}
// 线程函数
void *thread_func(void *args) {
int i, count = 0;
thread_args *thread_args = (thread_args *)args;
for (i = thread_args->start; i <= thread_args->end; i++) {
if (is_prime(i)) {
count++;
}
}
*(thread_args->count) += count;
pthread_exit(NULL);
}
int main() {
int i, count = 0;
pthread_t threads[NUM_THREADS];
thread_args args[NUM_THREADS];
// 分配线程任务
for (i = 0; i < NUM_THREADS; i++) {
args[i].start = RANGE_START + i*(RANGE_END-RANGE_START)/NUM_THREADS;
args[i].end = RANGE_START + (i+1)*(RANGE_END-RANGE_START)/NUM_THREADS - 1;
args[i].count = &count;
pthread_create(&threads[i], NULL, thread_func, (void *)&args[i]);
}
// 等待线程结束
for (i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
printf("Number of primes between %d and %d is %d\n", RANGE_START, RANGE_END, count);
return 0;
}
```
代码中使用了4个线程来计算,可以根据需要调整`NUM_THREADS`的值。线程函数`thread_func`中计算每个线程分配到的范围内的质数数量,并将结果累加到`count`变量中。最终输出计算结果。
阅读全文
相关推荐
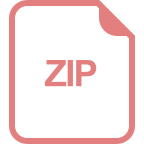
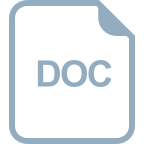

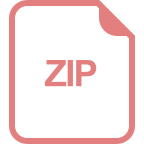




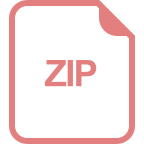
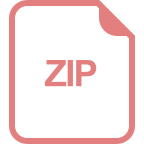
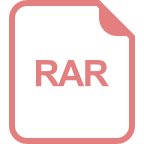
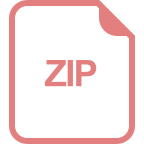
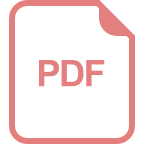
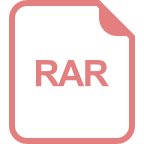
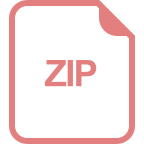
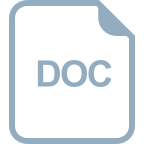
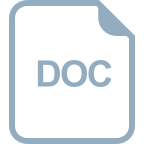