霍夫直线检测源代码,不使用opencv
时间: 2023-11-13 19:06:51 浏览: 89
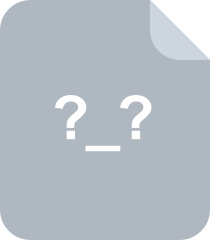
霍夫直线检测

霍夫直线检测是一种经典的图像处理算法,它可以在图像中检测出直线的位置和方向。以下是使用Python实现的简单霍夫直线检测算法,不使用OpenCV库。
```python
import numpy as np
import math
def hough_transform(img):
width, height = img.shape
theta_max = 180
rho_max = int(math.sqrt(width**2 + height**2))
accumulator = np.zeros((rho_max, theta_max), dtype=np.uint8)
for x in range(width):
for y in range(height):
if img[x][y] == 255:
for theta in range(theta_max):
rho = int(x * math.cos(theta * math.pi / 180) + y * math.sin(theta * math.pi / 180))
accumulator[rho][theta] += 1
return accumulator
def hough_lines(img, threshold):
accumulator = hough_transform(img)
width, height = accumulator.shape
lines = []
for rho in range(width):
for theta in range(height):
if accumulator[rho][theta] >= threshold:
a = math.cos(theta * math.pi / 180)
b = math.sin(theta * math.pi / 180)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * a)
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * a)
lines.append((x1, y1, x2, y2))
return lines
```
该代码包含两个函数:
1. `hough_transform`函数实现了霍夫变换,输入为二值化的图像,输出为霍夫空间中的累加器数组。
2. `hough_lines`函数使用霍夫变换结果,输入为二值化的图像和阈值,输出为所有检测到的直线的起始和结束点坐标。
需要注意的是,由于该实现没有使用OpenCV库,因此需要手动将图像转换为二值化格式。
阅读全文
相关推荐
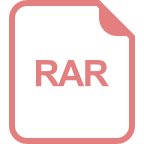
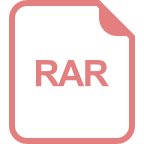
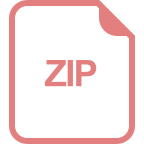
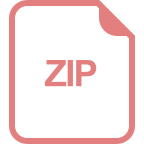
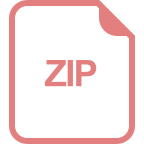
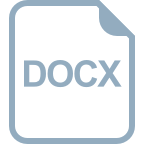
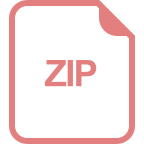
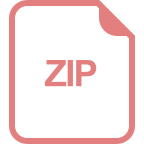
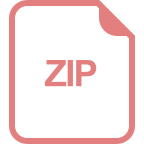
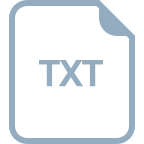
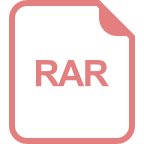
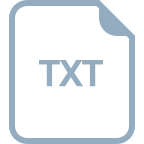
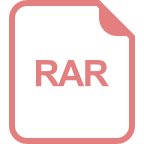
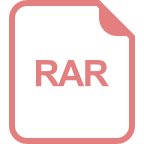
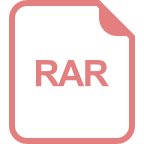