c语言编写2.给出n个学生的考试成绩表,每条信息由姓名和分数组成,试设计一个算法: (1)按分数高低次序,打印出每个学生在考试中获得的名次,分数相同的为同一名次; (2)按名次列出每个学生的姓名与分数。
时间: 2024-02-03 22:13:17 浏览: 59
这里给出两种算法实现:
算法一:
1. 首先读入n个学生的信息,存储在一个结构体数组中,包括姓名和分数。
2. 对每个学生的分数进行排序,得到一个分数数组。
3. 遍历每个学生,统计他的名次,即计算他的分数在分数数组中的位置。
4. 按照名次打印每个学生的信息,包括姓名、分数和名次。
算法二:
1. 首先读入n个学生的信息,存储在一个结构体数组中,包括姓名和分数。
2. 对结构体数组按照分数进行排序,得到一个按照分数从高到低排列的数组。
3. 遍历排好序的结构体数组,统计每个学生的名次,即当前分数与前一个分数不同的时候,当前名次加1。
4. 按照名次打印每个学生的信息,包括姓名、分数和名次。
以下是算法一的C语言代码实现:
```c
#include <stdio.h>
#include <string.h>
struct student {
char name[20];
int score;
};
int cmp(const void *a, const void *b) { // 用于qsort函数的比较函数
return ((struct student *) b)->score - ((struct student *) a)->score;
}
int main() {
int n;
struct student stu[100];
int i, j, rank;
int score[100];
printf("请输入学生人数:");
scanf("%d", &n);
for (i = 0; i < n; i++) {
printf("请输入第%d个学生的姓名和分数:", i + 1);
scanf("%s%d", stu[i].name, &stu[i].score);
score[i] = stu[i].score;
}
qsort(stu, n, sizeof(struct student), cmp);
printf("按分数高低次序,每个学生获得的名次如下:\n");
for (i = 0; i < n; i++) {
rank = 1;
for (j = 0; j < n; j++) {
if (stu[j].score > stu[i].score) {
rank++;
}
}
printf("%s的名次是%d\n", stu[i].name, rank);
}
printf("按名次列出每个学生的姓名和分数如下:\n");
for (i = 1; i <= n; i++) {
for (j = 0; j < n; j++) {
if (i == 1 && stu[j].score == score[0]) { // 处理第一名
printf("%d\t%s\t%d\n", i, stu[j].name, stu[j].score);
break;
}
if (stu[j].score == score[i - 1] && stu[j].score != score[i - 2]) {
printf("%d\t%s\t%d\n", i, stu[j].name, stu[j].score);
break;
}
}
}
return 0;
}
```
以下是算法二的C语言代码实现:
```c
#include <stdio.h>
#include <string.h>
struct student {
char name[20];
int score;
int rank;
};
int cmp(const void *a, const void *b) { // 用于qsort函数的比较函数
return ((struct student *) b)->score - ((struct student *) a)->score;
}
int main() {
int n;
struct student stu[100];
int i;
printf("请输入学生人数:");
scanf("%d", &n);
for (i = 0; i < n; i++) {
printf("请输入第%d个学生的姓名和分数:", i + 1);
scanf("%s%d", stu[i].name, &stu[i].score);
stu[i].rank = 0;
}
qsort(stu, n, sizeof(struct student), cmp);
stu[0].rank = 1;
for (i = 1; i < n; i++) {
if (stu[i].score == stu[i - 1].score) {
stu[i].rank = stu[i - 1].rank;
} else {
stu[i].rank = i + 1;
}
}
printf("按分数高低次序,每个学生获得的名次如下:\n");
for (i = 0; i < n; i++) {
printf("%s的名次是%d\n", stu[i].name, stu[i].rank);
}
printf("按名次列出每个学生的姓名和分数如下:\n");
for (i = 0; i < n; i++) {
printf("%d\t%s\t%d\n", stu[i].rank, stu[i].name, stu[i].score);
}
return 0;
}
```
阅读全文
相关推荐








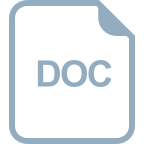









