android实现音乐播放器的代码和布局示例
时间: 2024-02-15 13:03:47 浏览: 27
以下是一个简单的Android音乐播放器的代码和布局示例:
1. 布局文件
在布局文件中,我们可以使用Android自带的播放器控件`MediaPlayer`来实现音乐播放器的UI界面。以下是一个简单的布局文件示例:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<VideoView
android:id="@+id/videoView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<LinearLayout
android:id="@+id/controls"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:orientation="horizontal"
android:padding="16dp">
<Button
android:id="@+id/button_play"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Play" />
<Button
android:id="@+id/button_pause"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Pause" />
<SeekBar
android:id="@+id/seekBar"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:max="100"
android:paddingLeft="16dp"
android:paddingRight="16dp" />
</LinearLayout>
</RelativeLayout>
```
在上述布局文件中,我们首先使用`RelativeLayout`作为根布局,并在其中添加了一个`VideoView`控件作为播放器的UI界面。接下来,我们在底部添加了一个`LinearLayout`控件,用于放置播放控制按钮和进度条。其中,`SeekBar`控件用于显示播放进度,`Button`控件用于实现播放、暂停操作。
2. Java代码
在Java代码中,我们需要实现播放控制按钮的点击事件,以及在`SeekBar`控件上显示播放进度。以下是一个简单的Java代码示例:
```java
public class MainActivity extends AppCompatActivity {
private VideoView videoView;
private Button buttonPlay;
private Button buttonPause;
private SeekBar seekBar;
private int totalTime;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
videoView = findViewById(R.id.videoView);
buttonPlay = findViewById(R.id.button_play);
buttonPause = findViewById(R.id.button_pause);
seekBar = findViewById(R.id.seekBar);
// 设置播放器的视频资源
videoView.setVideoURI(Uri.parse("android.resource://" + getPackageName() + "/" + R.raw.sample));
// 点击播放按钮开始播放
buttonPlay.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
videoView.start();
}
});
// 点击暂停按钮暂停播放
buttonPause.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
videoView.pause();
}
});
// 显示播放进度
videoView.setOnPreparedListener(new MediaPlayer.OnPreparedListener() {
@Override
public void onPrepared(MediaPlayer mediaPlayer) {
totalTime = mediaPlayer.getDuration();
seekBar.setMax(totalTime);
}
});
videoView.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mediaPlayer) {
videoView.seekTo(0);
buttonPlay.setText("Play");
}
});
final Handler handler = new Handler();
Runnable runnable = new Runnable() {
@Override
public void run() {
int currentPosition = videoView.getCurrentPosition();
seekBar.setProgress(currentPosition);
handler.postDelayed(this, 1000);
}
};
handler.postDelayed(runnable, 1000);
}
}
```
在上述Java代码中,我们首先通过`findViewById`方法获取了布局文件中的控件对象。接着,我们使用`setVideoURI`方法设置了播放器的视频资源。然后,我们实现了播放控制按钮的点击事件,通过调用`start`方法开始播放,通过调用`pause`方法暂停播放。在`SeekBar`控件上,我们通过`setOnPreparedListener`方法获取了视频的总时长,并将其设置为`SeekBar`的最大值。在播放过程中,我们通过`getCurrentPosition`方法获取当前播放位置,并将其设置为`SeekBar`的进度。最后,我们使用`Handler`和`Runnable`实现了每秒更新一次播放进度的操作。
以上是一个简单的Android音乐播放器的代码和布局示例。需要注意的是,以上示例代码仅供参考,实际情况中可能需要根据具体需求进行修改和优化。
相关推荐
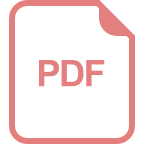
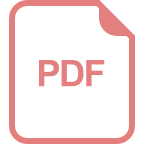
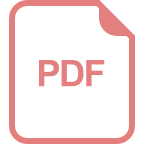














