python如何读取数据量大的sqlite3数据库并且不会造成内存溢出,并且采用多线程速度提取
时间: 2024-03-17 13:41:18 浏览: 217
要读取数据量大的SQLite3数据库并且不会造成内存溢出,并且采用多线程速度提取,可以考虑使用以下技术:
1. 使用Python内置的sqlite3模块连接到SQLite3数据库,使用fetchmany()方法分批次从数据库中提取数据,避免一次性读取过多数据导致内存溢出。
2. 使用Python的multiprocessing模块实现多线程处理,加速数据提取和处理过程。
示例代码:
```python
import sqlite3
import multiprocessing as mp
# 每次从数据库中读取的行数
BATCH_SIZE = 10000
# 数据处理函数
def process_data(data):
# 处理数据的代码
pass
# 多线程处理函数
def process_batch(conn, query, offset):
# 连接到数据库
conn = sqlite3.connect(conn)
# 读取数据
cursor = conn.cursor()
cursor.execute(query + ' LIMIT ? OFFSET ?', (BATCH_SIZE, offset))
data = cursor.fetchall()
# 处理数据
process_data(data)
# 关闭数据库连接
cursor.close()
conn.close()
# 主函数
def main():
# 连接到SQLite3数据库
conn = sqlite3.connect('database.db')
# 查询语句
query = 'SELECT * FROM table_name'
# 获取数据总行数
cursor = conn.cursor()
cursor.execute(query)
total_rows = cursor.fetchone()[0]
cursor.close()
# 创建进程池
pool = mp.Pool()
# 分批次处理数据
for offset in range(0, total_rows, BATCH_SIZE):
pool.apply_async(process_batch, args=(conn, query, offset))
# 等待所有线程完成
pool.close()
pool.join()
# 关闭数据库连接
conn.close()
if __name__ == '__main__':
main()
```
请注意,这只是一个示例,具体的实现取决于数据量的大小和计算机的性能。如果处理的数据量非常大,您可能需要考虑使用其他技术来优化性能,如使用多台计算机并行处理、使用数据库索引等。同时,还需要注意线程安全和数据一致性问题。
阅读全文
相关推荐
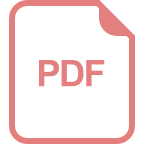
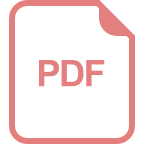
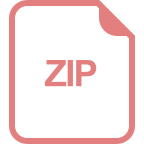



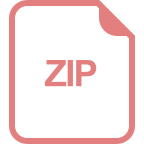
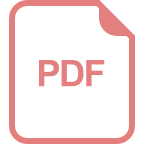
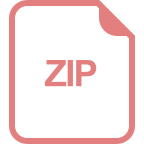
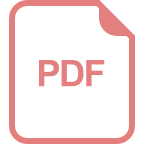
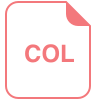
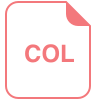
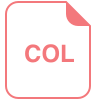
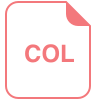
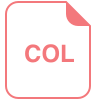
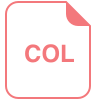
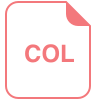
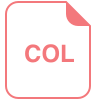
