uniapp 模拟点击屏幕
时间: 2023-10-29 21:05:33 浏览: 529
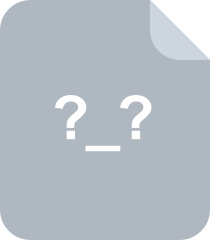
安卓手机,模拟屏幕点击 免费版
可以使用uniapp的原生事件模拟方法来实现点击屏幕的效果。具体步骤如下:
1. 获取需要点击的元素的dom节点
2. 创建一个MouseEvent对象,设置其type为'click',并且设置其坐标位置
3. 调用dom节点的dispatchEvent方法,触发模拟点击事件
以下是示例代码:
```javascript
// 获取需要点击的元素
const target = document.querySelector('.target')
// 创建MouseEvent对象
const event = new MouseEvent('click', {
view: window,
bubbles: true,
cancelable: true,
clientX: target.offsetLeft + target.offsetWidth / 2,
clientY: target.offsetTop + target.offsetHeight / 2
})
// 触发模拟点击事件
target.dispatchEvent(event)
```
阅读全文
相关推荐
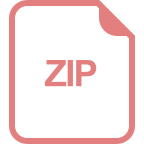
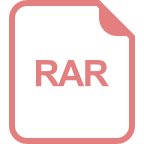
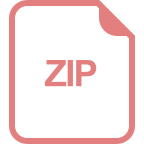
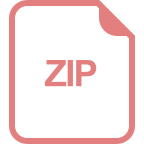
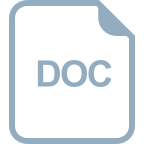
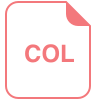


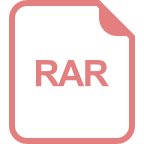
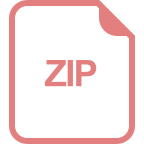
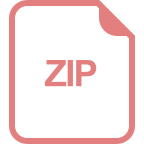
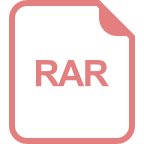
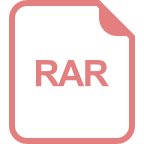
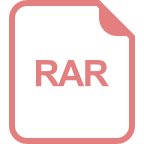
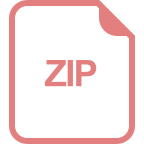
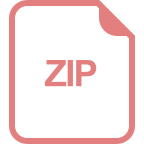
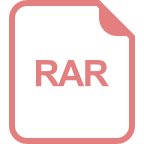