python tkinter实现通过输入鼠标在电脑上坐标位置x,y,以及左右键单击或双击,通过时间顺序,使系统依次读取输入的鼠标操作,通过pyautogui实现自动化。
时间: 2023-12-03 11:42:58 浏览: 74
好的,下面是实现这个功能的Python代码:
```python
import tkinter as tk
import pyautogui
# 创建主窗口
root = tk.Tk()
root.title("鼠标操作记录")
# 创建文本框
text = tk.Text(root, width=50, height=20)
text.pack()
# 记录鼠标操作的列表
mouse_actions = []
# 鼠标操作记录函数
def record_mouse_action(event):
# 获取鼠标位置和操作类型
x, y = event.x, event.y
action_type = "left_double_click" if event.num == 1 and event.type == "ButtonRelease" and event.num == event.num else \
"right_double_click" if event.num == 3 and event.type == "ButtonRelease" and event.num == event.num else \
"left_click" if event.num == 1 and event.type == "ButtonRelease" else \
"right_click" if event.num == 3 and event.type == "ButtonRelease" else None
if action_type:
# 在文本框中显示鼠标操作
text.insert(tk.END, f"{action_type} at ({x}, {y})\n")
# 添加鼠标操作到列表中
mouse_actions.append((action_type, x, y))
# 绑定鼠标事件
root.bind("<ButtonRelease>", record_mouse_action)
# 执行鼠标操作函数
def perform_mouse_actions():
for action in mouse_actions:
# 获取鼠标操作类型和位置
action_type, x, y = action
# 执行鼠标操作
if action_type == "left_click":
pyautogui.click(x=x, y=y, button="left")
elif action_type == "right_click":
pyautogui.click(x=x, y=y, button="right")
elif action_type == "left_double_click":
pyautogui.doubleClick(x=x, y=y, button="left")
elif action_type == "right_double_click":
pyautogui.doubleClick(x=x, y=y, button="right")
# 创建执行按钮
button = tk.Button(root, text="执行鼠标操作", command=perform_mouse_actions)
button.pack()
# 运行主程序
root.mainloop()
```
这个程序创建了一个窗口,可以在窗口中记录鼠标操作,然后点击“执行鼠标操作”按钮可以自动执行鼠标操作。程序使用了Python的tkinter模块创建窗口,使用了pyautogui模块执行鼠标操作。
阅读全文
相关推荐
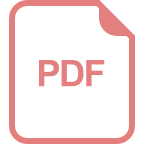
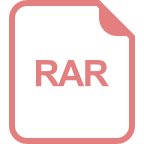
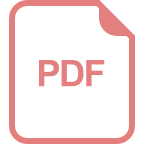
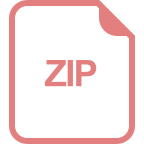
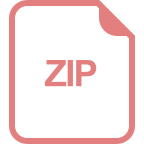
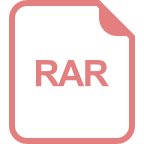
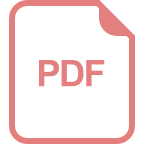
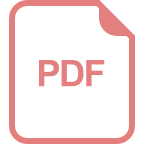
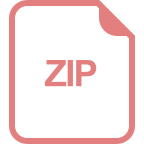
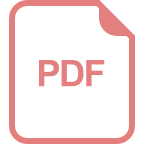
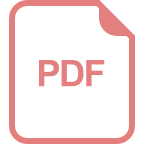
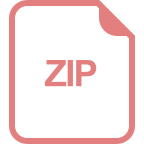
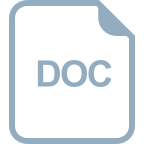
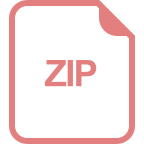
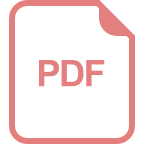