pytest request allure
时间: 2023-06-05 19:47:15 浏览: 171
pytest是一个Python测试框架,可以用于编写和运行各种类型的测试,包括单元测试、集成测试和功能测试等。request是pytest框架中的一个插件,可以用于发送HTTP请求和处理响应。allure是一个测试报告生成工具,可以将pytest框架生成的测试结果转换为漂亮的HTML报告,方便测试人员查看和分析测试结果。
相关问题
Python+request+pytest+allure核心代码
以下是一个使用Python + requests + pytest + allure的核心代码示例:
```python
import requests
import pytest
import allure
@allure.feature("接口测试")
class TestAPI:
@allure.story("测试接口1")
def test_api1(self):
url = "http://api.example.com/api1"
headers = {"Content-Type": "application/json"}
data = {"key": "value"}
response = requests.post(url, headers=headers, json=data)
assert response.status_code == 200
assert response.json()["result"] == "success"
allure.attach(name="请求参数", body=str(data), attachment_type=allure.attachment_type.JSON)
allure.attach(name="响应结果", body=str(response.json()), attachment_type=allure.attachment_type.JSON)
@allure.story("测试接口2")
def test_api2(self):
url = "http://api.example.com/api2"
headers = {"Content-Type": "application/json"}
data = {"key": "value"}
response = requests.post(url, headers=headers, json=data)
assert response.status_code == 200
assert response.json()["result"] == "success"
allure.attach(name="请求参数", body=str(data), attachment_type=allure.attachment_type.JSON)
allure.attach(name="响应结果", body=str(response.json()), attachment_type=allure.attachment_type.JSON)
if __name__ == "__main__":
pytest.main(["-s", "-v", "--alluredir", "./allure-results"])
```
这段代码演示了如何使用Python的requests库发送HTTP请求,并结合pytest和allure进行接口测试。其中,`test_api1`和`test_api2`是两个测试用例,分别发送POST请求到不同的接口,并对响应结果进行断言。使用allure的`attach`方法可以将请求参数和响应结果作为附件展示在测试报告中。
搭建一个pytest+allure+request接口自动化框架
好的,这里为您提供一个简单的pytest+allure+requests接口自动化框架搭建步骤:
1. 安装pytest和allure
```
pip install pytest
pip install allure-pytest
```
2. 安装requests库
```
pip install requests
```
3. 创建测试用例文件和测试数据文件
在项目中创建一个tests文件夹,用于存放测试用例文件。在tests文件夹中创建一个test_api.py文件,用于编写测试用例。
同时,也需要在项目中创建一个data文件夹,用于存放测试数据文件。在data文件夹中创建一个api_data.json文件,用于存放接口测试数据。
4. 编写测试用例
在test_api.py文件中,使用pytest编写测试用例。可以使用requests库发送接口请求,并对返回结果进行断言。
示例代码:
```python
import pytest
import requests
class TestAPI:
@pytest.fixture(scope='session', autouse=True)
def setup_class(self):
self.base_url = 'https://api.github.com'
def test_get_user(self):
url = self.base_url + '/users/github'
response = requests.get(url)
assert response.status_code == 200
assert response.json()['login'] == 'github'
def test_create_gist(self):
url = self.base_url + '/gists'
data = {
"description": "test gist",
"public": True,
"files": {
"test.txt": {
"content": "Test gist content"
}
}
}
headers = {
"Authorization": "token <your access token>"
}
response = requests.post(url, json=data, headers=headers)
assert response.status_code == 201
```
5. 编写测试数据
在api_data.json文件中,编写接口测试用到的数据。可以对不同接口的测试数据进行分类,方便维护。
示例代码:
```json
{
"get_user": {
"url": "/users/github",
"method": "get",
"headers": {},
"params": {},
"data": {},
"json": {},
"expected_status_code": 200,
"expected_data": {
"login": "github"
}
},
"create_gist": {
"url": "/gists",
"method": "post",
"headers": {
"Authorization": "token <your access token>"
},
"params": {},
"data": {
"description": "test gist",
"public": true,
"files": {
"test.txt": {
"content": "Test gist content"
}
}
},
"json": {},
"expected_status_code": 201,
"expected_data": {}
}
}
```
6. 使用pytest执行测试用例
在项目根目录下打开终端,执行如下命令:
```
pytest --alluredir=./report
```
该命令将会执行tests文件夹下的所有测试用例,并将测试结果保存在report文件夹中。
7. 生成测试报告
执行完测试用例后,使用如下命令生成测试报告:
```
allure generate ./report -o ./report/html --clean
```
该命令将会在report文件夹下生成一个html文件夹,里面包含测试结果的详细信息和图表。
至此,一个简单的pytest+allure+requests接口自动化框架就搭建完成了。
阅读全文
相关推荐
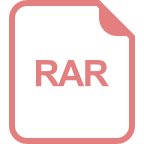
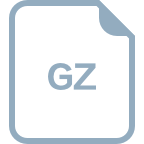
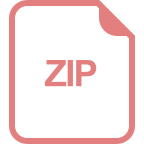
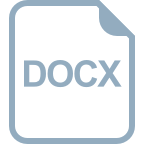


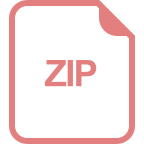
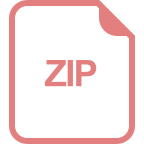
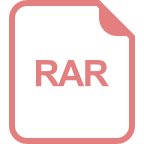
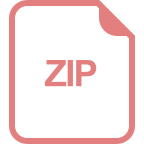
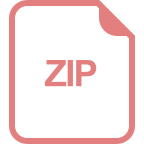
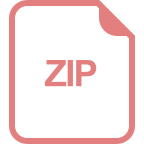
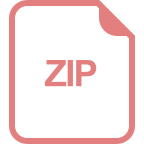
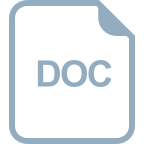

