antd react 固定表头
时间: 2023-07-01 13:18:31 浏览: 112
如果你使用的是 antd 的 Table 组件,那么可以通过设置 `scroll` 属性来实现固定表头。具体实现方法如下:
1. 设置 `scroll` 属性为一个对象,包含两个属性 `x` 和 `y`,分别表示横向和纵向滚动条的配置。
```jsx
<Table
columns={columns}
dataSource={dataSource}
scroll={{ x: 1500, y: 300 }}
/>
```
2. 设置 `columns` 的 `fixed` 属性为 `'left'` 或 `'right'`,表示该列固定在左侧或右侧。
```jsx
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
fixed: 'left', // 固定在左侧
width: 100,
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
width: 100,
},
// 其他列
];
```
注意,如果设置了 `scroll` 属性,那么表格宽度必须大于 `scroll.x` 才能显示横向滚动条。同时,固定列的总宽度也不能超过表格宽度。
相关问题
antd react 虚拟列表怎么固定表头
antd 的虚拟列表组件是 `List`,而不是 `Table`,它不支持固定表头。不过,你可以自己实现一个带有固定表头的虚拟列表组件。具体实现方法如下:
1. 使用 `List` 组件渲染数据,并设置 `itemCount` 和 `itemSize` 属性,分别表示数据项的总数和每个数据项的高度。
```jsx
import { List } from 'antd';
function VirtualTable({ columns, dataSource, height }) {
const itemSize = 50; // 每个数据项的高度
const itemCount = dataSource.length; // 数据项的总数
return (
<List
className="virtual-table"
itemLayout="horizontal"
dataSource={dataSource}
renderItem={(item, index) => (
<List.Item>
<List.Item.Meta
title={item.name}
description={item.age}
/>
{/* 其他列 */}
</List.Item>
)}
height={height}
itemSize={itemSize}
itemCount={itemCount}
/>
);
}
```
2. 在列表的外层包裹一个 `div` 元素,设置该元素的宽度和高度,并设置 `overflow: auto`,使其出现滚动条。
```jsx
function VirtualTable({ columns, dataSource, height }) {
// ...
return (
<div className="virtual-table-container" style={{ height: height }}>
<List
// ...
/>
</div>
);
}
```
3. 在 `renderItem` 中增加一个固定表头的 `div` 元素,设置该元素的宽度和高度,并设置 `position: sticky`,使其跟随滚动条滚动时固定在顶部。
```jsx
function VirtualTable({ columns, dataSource, height }) {
// ...
return (
<div className="virtual-table-container" style={{ height: height }}>
<div className="virtual-table-header">
<List.Item>
{columns.map((column, index) => (
<List.Item.Meta
key={index}
title={column.title}
description={column.dataIndex}
style={{ width: column.width }}
/>
))}
</List.Item>
</div>
<List
// ...
/>
</div>
);
}
```
4. 在 CSS 中设置样式,使表头和数据项对齐。
```css
.virtual-table-header {
position: sticky;
top: 0;
background-color: #fff;
z-index: 1;
}
.virtual-table .ant-list-item-meta-description {
line-height: 50px; /* 每个数据项的高度 */
}
```
完整代码如下:
```jsx
import { List } from 'antd';
import './VirtualTable.css';
function VirtualTable({ columns, dataSource, height }) {
const itemSize = 50; // 每个数据项的高度
const itemCount = dataSource.length; // 数据项的总数
return (
<div className="virtual-table-container" style={{ height: height }}>
<div className="virtual-table-header">
<List.Item>
{columns.map((column, index) => (
<List.Item.Meta
key={index}
title={column.title}
description={column.dataIndex}
style={{ width: column.width }}
/>
))}
</List.Item>
</div>
<List
className="virtual-table"
itemLayout="horizontal"
dataSource={dataSource}
renderItem={(item, index) => (
<List.Item>
<List.Item.Meta
title={item.name}
description={item.age}
/>
{/* 其他列 */}
</List.Item>
)}
height={height}
itemSize={itemSize}
itemCount={itemCount}
/>
</div>
);
}
```
```css
.virtual-table-container {
width: 100%;
overflow: auto;
}
.virtual-table-header {
position: sticky;
top: 0;
background-color: #fff;
z-index: 1;
}
.virtual-table .ant-list-item-meta-description {
line-height: 50px; /* 每个数据项的高度 */
}
```
antd 设置表头属性,使用表数据自定义React Antd表头
如果要使用表数据自定义React Antd表头,可以使用Antd Table组件的render函数来自定义表头。
首先,你需要定义一个表格数据源,例如:
```
const dataSource = [
{
key: '1',
name: 'John Brown',
age: 32,
address: 'New York No. 1 Lake Park',
},
{
key: '2',
name: 'Jim Green',
age: 42,
address: 'London No. 1 Lake Park',
},
{
key: '3',
name: 'Joe Black',
age: 32,
address: 'Sidney No. 1 Lake Park',
},
];
```
然后,你可以使用Table组件的columns属性来定义表头。在columns中,可以使用render函数来自定义表头的展示方式,例如:
```
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
render: (text, record) => (
<span>
{text} - {record.address}
</span>
),
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
},
{
title: 'Address',
dataIndex: 'address',
key: 'address',
},
];
```
在上面的代码中,我们使用render函数来自定义了Name列的展示方式,将姓名和地址连接起来展示。
最后,将dataSource和columns作为Table组件的属性传入即可自定义Antd表头。例如:
```
<Table dataSource={dataSource} columns={columns} />
```
阅读全文
相关推荐
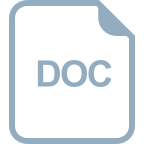
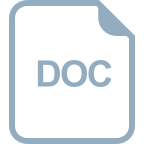
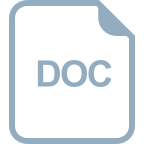
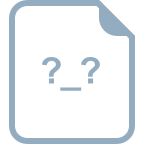

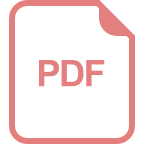

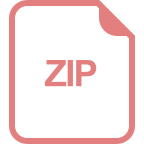








