python 使用bs4判断网页的标签是否包含某标签
时间: 2023-08-02 12:06:20 浏览: 308
你可以使用BeautifulSoup4库中的find方法来查找标签,如果找到则返回该标签,否则返回None。以下是一个示例代码,可以判断网页中是否包含某个标签:
```python
from bs4 import BeautifulSoup
import requests
# 获取网页内容
url = 'https://www.example.com'
response = requests.get(url)
html = response.content
# 创建BeautifulSoup对象
soup = BeautifulSoup(html, 'html.parser')
# 查找标签
tag = soup.find('div', {'class': 'example-class'})
# 判断是否找到标签
if tag is not None:
print('找到了该标签')
else:
print('未找到该标签')
```
在上面的代码中,我们首先使用requests库获取网页内容,然后使用BeautifulSoup库创建一个BeautifulSoup对象。接着,我们使用find方法查找class为example-class的div标签,并将结果保存在变量tag中。最后,我们判断tag是否为None,如果不是则说明找到了该标签。
相关问题
python 使用判断某一个的标签是否包含某标签代码
你可以使用 BeautifulSoup 库中的 `find` 或 `find_all` 方法来查找 HTML 标签,并使用 `has_attr` 方法来判断是否包含某个属性。
下面是一个例子:
```python
from bs4 import BeautifulSoup
html = '''
<html>
<head>
<title>这是标题</title>
</head>
<body>
<div class="content">
<p>这是正文</p>
</div>
</body>
</html>
'''
soup = BeautifulSoup(html, 'html.parser')
# 查找 div 标签,并判断是否包含 class 属性
div_tag = soup.find('div')
if div_tag.has_attr('class'):
print('div 标签包含 class 属性')
else:
print('div 标签没有包含 class 属性')
# 查找 p 标签,并判断是否包含 id 属性
p_tags = soup.find_all('p')
for p_tag in p_tags:
if p_tag.has_attr('id'):
print('p 标签包含 id 属性')
else:
print('p 标签没有包含 id 属性')
```
输出结果:
```
div 标签包含 class 属性
p 标签没有包含 id 属性
```
python在bs4中rel的用法
在Python的BeautifulSoup库中,我们可以通过以下方式来获取HTML标签的rel属性值:
1. 找到对应的HTML标签对象,可以使用find()、find_all()等方法来查找。
```
tag = soup.find('a', href='http://example.com')
```
2. 使用get()方法获取rel属性值:
```
rel_value = tag.get('rel')
```
如果该标签没有rel属性,则返回None。
如果一个标签有多个rel属性值,可以通过以下方式获取到所有的值:
```
rel_values = tag.get('rel', [])
```
此时,rel_values为一个列表,包含了所有的rel属性值。
如果需要判断某个值是否在rel属性中,可以使用in关键字:
```
if 'stylesheet' in rel_values:
...
```
除了使用get()方法,我们还可以通过直接访问标签对象的属性来获取rel属性值:
```
rel_value = tag['rel']
```
但是如果该标签没有rel属性,这种方式会抛出KeyError异常,因此在使用时需要注意。
阅读全文
相关推荐
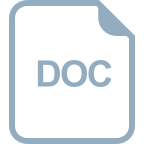
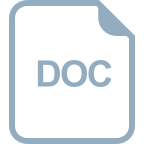
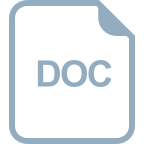
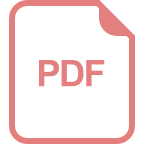
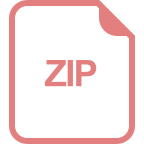
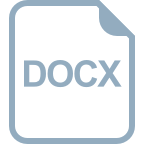
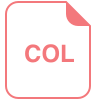
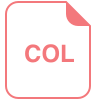
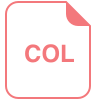
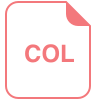
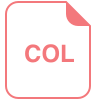
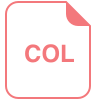
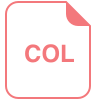
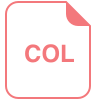
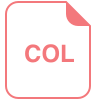
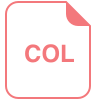
