Java中JSONarray转成JSONobject的常用的3种方法
时间: 2023-12-14 09:37:32 浏览: 485
1. 使用循环遍历JSONArray,将每个JSONObject添加到新的JSONObject中。
```java
JSONArray jsonArray = new JSONArray();
JSONObject jsonObject = new JSONObject();
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject object = jsonArray.getJSONObject(i);
jsonObject.put(String.valueOf(i), object);
}
```
2. 将JSONArray转成String,再使用JSONObject解析String。
```java
JSONArray jsonArray = new JSONArray();
JSONObject jsonObject = new JSONObject(jsonArray.toString());
```
3. 使用GSON库进行转换。
```java
JSONArray jsonArray = new JSONArray();
Gson gson = new Gson();
JSONObject jsonObject = new JSONObject(gson.toJson(jsonArray));
```
注意:在使用第三种方法之前,需要先在项目中导入GSON库。
相关问题
Java中给你一串string数据要求先转成JSONarray再转成JSONobject要求最后的格式是[{{}]
首先,需要使用JSON库来完成这个任务,比如常用的JSON库有Gson、Jackson等。下面是使用Gson库的示例代码:
```java
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import com.google.gson.JsonObject;
public class StringToJson {
public static void main(String[] args) {
String str = "[{\"name\":\"Tom\",\"age\":20},{\"name\":\"Jerry\",\"age\":18}]";
Gson gson = new Gson();
JsonArray jsonArray = gson.fromJson(str, JsonArray.class);
JsonObject jsonObject = new JsonObject();
jsonObject.add("", jsonArray);
String result = "[" + jsonObject.toString() + "]";
System.out.println(result);
}
}
```
在这段代码中,首先将输入的字符串转换成JsonArray对象,然后将该对象添加到一个新的JsonObject中,这里使用了一个空字符串""作为JsonObject的key。最后将该JsonObject转换为字符串并添加上外层的中括号,就得到了要求的结果。
java多次判断JSONObject和JSONArray
在Java中,你可以使用`org.json.JSONObject`和`org.json.JSONArray`来处理JSON数据,包括多次判断它们的内容。这里以`Fastjson`, `Gson`, 和 `org.json`这三种常用的JSON库为例[^1]:
1. **Fastjson**[^1]: Fastjson提供了便捷的方法来判断。例如,检查一个JSONObject是否有某个键(key):
```java
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "John");
boolean hasName = jsonObject.containsKey("name"); // 判断是否存在"name"键
System.out.println(hasName); // 输出:true 或 false
```
2. **Gson**: GSON库也提供了相似的功能。检查一个JSONObject同样可以使用containsKey:
```java
Gson gson = new Gson();
JsonObject jsonObject = gson.fromJson("{\"name\":\"John\"}", JsonObject.class);
boolean hasName = jsonObject.has("name"); // 判读存在性
System.out.println(hasName); // 输出:true 或 false
```
3. **org.json**: 对于org.json库,你需要通过`.optBoolean(key)`、`.optInt(key)`等方法间接判断其类型,如:
```java
import org.json.JSONObject;
JSONObject jsonArray = new JSONObject("[{ \"name\": \"John\", \"age\": 30 }]");
boolean hasName = jsonArray.getJSONObject(0).has("name"); // 检查第一个元素是否有"name"
System.out.println(hasName); // 输出:true
```
4. **JSONArray**: JSONArray的判断通常涉及到数组长度和索引访问。比如:
```java
JSONArray jsonArray = new JSONArray("[\"Apple\", \"Banana\", \"Cherry\"]");
if (jsonArray.length() > 0) { // 判断非空
String firstFruit = jsonArray.getString(0); // 获取第一个元素
System.out.println(firstFruit); // 输出:"Apple"
}
```
阅读全文
相关推荐
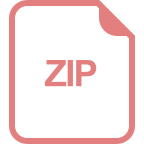
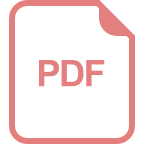
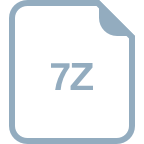
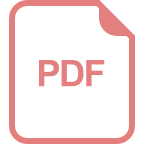
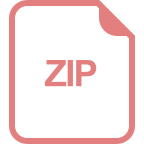
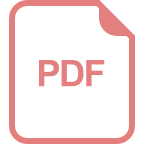
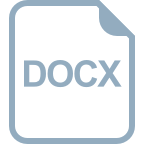
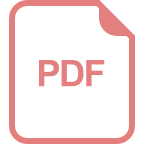
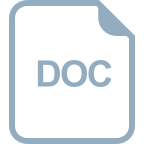






