c++用max两两求四个数最大
时间: 2024-03-16 22:46:14 浏览: 59
你可以使用 C++ 中的 `max` 函数来求四个数中的最大值。可以先两两比较,然后再将得到的两个最大值进行比较,得出最终的最大值。
以下是示例代码:
```cpp
int a = 10, b = 20, c = 30, d = 40;
int max1 = max(a, b); // 比较 a 和 b,得到其中的最大值
int max2 = max(c, d); // 比较 c 和 d,得到其中的最大值
int max_final = max(max1, max2); // 比较 max1 和 max2,得到最终的最大值
cout << "四个数中的最大值为:" << max_final << endl;
```
输出结果为:
```
四个数中的最大值为:40
```
相关问题
c++求四个数的最大数
你可以使用条件语句来求解四个数的最大值。以下是一个使用C++编写的示例代码:
```cpp
#include <iostream>
int main() {
int a, b, c, d;
std::cout << "请输入四个数:" << std::endl;
std::cin >> a >> b >> c >> d;
int max = a;
if (b > max) {
max = b;
}
if (c > max) {
max = c;
}
if (d > max) {
max = d;
}
std::cout << "最大数为:" << max << std::endl;
return 0;
}
```
在这个示例中,我们通过比较四个数与当前最大值,逐步更新最大值,最后输出结果。
用c++求两个数的最大公约数
以下是两种用C++求两个数的最大公约数的方法:
方法一:暴力法
```cpp
#include <iostream>
using namespace std;
int main() {
int m, n, gcd;
cin >> m >> n;
gcd = m > n ? n : m;
while (gcd > 1 && (m % gcd != 0 || n % gcd != 0)) {
gcd--;
}
cout << gcd << endl;
return 0;
}
```
方法二:欧几里德算法(辗转相除法)
```cpp
#include <iostream>
using namespace std;
int main() {
int m, n;
cin >> m >> n; int r = m % n;
while (r != 0) {
m = n;
n = r;
r = m % n;
}
cout << "gcd=" << n << endl;
return 0;
}
```
相关推荐
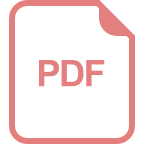












