audit.vue:1993 Uncaught TypeError: Cannot read properties of undefined (reading '$axios') at eval
时间: 2023-11-19 16:57:15 浏览: 187
这个错误通常是因为在Vue.js项目中没有正确引入$axios导致的。$axios是Vue.js中的一个插件,用于发送HTTP请求。要解决这个问题,您可以按照以下步骤进行操作:
1. 确保您已经正确安装了$axios插件。您可以在项目的main.js文件中添加以下代码来安装它:
```javascript
import Vue from 'vue'
import axios from 'axios'
import VueAxios from 'vue-axios'
Vue.use(VueAxios, axios)
```
2. 确保您已经正确引入了$axios。您可以在您的组件中添加以下代码来引入它:
```javascript
import axios from 'axios'
export default {
name: 'YourComponent',
mounted () {
axios.get('/your-api-endpoint')
.then(response => {
console.log(response.data)
})
}
}
```
3. 如果您已经正确安装和引入了$axios,但仍然遇到这个错误,请检查您的代码是否有其他问题,例如拼写错误或语法错误。
相关问题
PageSpeed 报错axe-core Error: Cannot read properties of undefined (reading 'getCellFromPoint')
PageSpeed 是一种由 Google 开发的工具,用于评估网页性能并提供优化建议。它通常与 Lighthouse 或 Chrome DevTools 的 Audit 部分一起使用。"axe-core" 是 axe 的核心模块,这是一个无障碍审计库,用于检查网站是否符合 Web Content Accessibility Guidelines (WCAG)。
错误 "Cannot read properties of undefined (reading 'getCellFromPoint')" 意味着在执行某个 axe 的检查时,遇到了一个 `undefined` 对象,并试图访问其名为 'getCellFromPoint' 的属性,这通常是由于尝试获取表格或其他元素的位置信息,但在当前页面或上下文中找不到这个元素。
解决这个问题需要定位到具体的源码行,可能是某个依赖库未正确加载、DOM 结构发生了变化,或者在某些特定条件下该功能不可用。常见的排查步骤包括:
1. 检查是否有误引用的库或函数,确认 'getCellFromPoint' 是否应该存在。
2. 确保页面上存在 axe 执行此操作所需的元素。
3. 使用浏览器开发者工具查看错误发生的上下文,看看是否存在渲染问题或元素缺失的情况。
4. 如果是在自动化测试环境中,确保模拟用户交互的行为设置正确。
解释:Error flushing statements. Cause: org.sqlite.SQLiteException: [SQLITE_CONSTRAINT_PRIMARYKEY] A PRIMARY KEY constraint failed (UNIQUE constraint failed: AUDIT_SCORE_INTE_DATA_VAR.DATAINTE_VAR_ID) ### Cause: org.sqlite.SQLiteException: [SQLITE_CONSTRAINT_PRIMARYKEY] A PRIMARY KEY constraint failed (UNIQUE constraint failed: AUDIT_SCORE_INTE_DATA_VAR.DATAINTE_VAR_ID) ; uncategorized SQLException; SQL state [null]; error code [19]; [SQLITE_CONSTRAINT_PRIMARYKEY] A PRIMARY KEY constraint failed (UNIQUE constraint failed: AUDIT_SCORE_INTE_DATA_VAR.DATAINTE_VAR_ID); nested exception is org.sqlite.SQLiteException: [SQLITE_CONSTRAINT_PRIMARYKEY] A PRIMARY KEY constraint failed (UNIQUE constraint failed: AUDIT_SCORE_INTE_DATA_VAR.DATAINTE_VAR_ID)
这个错误是因为在执行 SQL 语句时,试图插入一个已经存在的主键,从而导致唯一性约束失败。具体来说,这个错误是由于在插入 AUDIT_SCORE_INTE_DATA_VAR 表中的数据时,试图插入一个已经存在的 DATAINTE_VAR_ID 主键所引起的。这个错误代码是 SQLITE_CONSTRAINT_PRIMARYKEY,表示主键约束失败。
阅读全文
相关推荐
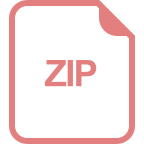
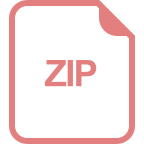
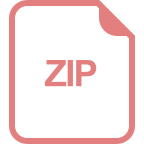
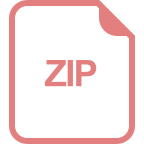
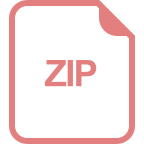
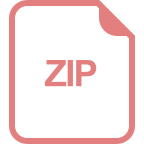
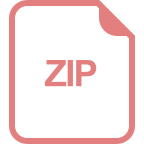
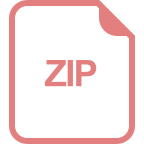
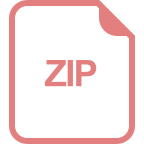
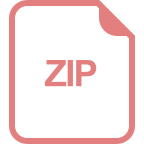
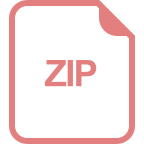
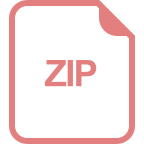
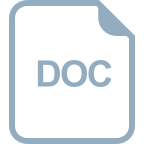




