使用python Tkinter建立一个窗口,添加“选择源路径1”,添加“选择目标路径1”,添加“选择源路径2”,添加“选择目标路径2”,添加“选择源路径3”,添加“选择目标路径3”,添加“选择源路径4”,添加“选择目标路径4”,添加“选择源路径5”,添加“选择目标路径5”,建立一个“开始执行”按钮,建立一个“取消执行”按钮,当点击“开始按钮”后用1000个线程遍历源路径下的文件是否已经在目标路径下存在,因为源路径和目标路径有几亿个文件,并用CRC校验源路径和目标路径的文件CRC值是否相符,如果校验值不相符则拷贝,否则不拷贝,要求程序24小时运行监控如果检测源路径有新文件产生就立刻拷贝到目标路径,程序在运行时“开始按钮”为灰色。当点击“取消执行”按钮后程序停止运行。
时间: 2023-12-15 15:04:04 浏览: 25
好的,以下是代码实现:
```python
import tkinter as tk
import threading
import os
import shutil
import zlib
class FileCopyThread(threading.Thread):
"""
文件复制线程类
"""
def __init__(self, src_path, dst_path):
super().__init__()
self.src_path = src_path
self.dst_path = dst_path
def run(self):
# 判断源文件是否存在于目标路径中,如果不存在则进行复制
if not os.path.exists(os.path.join(self.dst_path, os.path.basename(self.src_path))):
# 计算源文件的CRC值
with open(self.src_path, 'rb') as f:
src_crc = zlib.crc32(f.read())
# 计算目标路径中同名文件的CRC值
dst_file_path = os.path.join(self.dst_path, os.path.basename(self.src_path))
if os.path.exists(dst_file_path):
with open(dst_file_path, 'rb') as f:
dst_crc = zlib.crc32(f.read())
else:
dst_crc = -1
# 如果CRC值不相等,则进行复制
if src_crc != dst_crc:
shutil.copy(self.src_path, self.dst_path)
class App:
def __init__(self, root):
self.root = root
self.root.title("文件复制工具")
self.file_path_var = []
self.thread_list = []
self.create_widgets()
self.root.mainloop()
def create_widgets(self):
# 添加“选择源路径1”按钮
self.src_path_btn1 = tk.Button(self.root, text="选择源路径1", command=lambda:self.select_file_path(0))
self.src_path_btn1.grid(row=0, column=0, padx=5, pady=5)
# 添加“选择目标路径1”按钮
self.dst_path_btn1 = tk.Button(self.root, text="选择目标路径1", command=lambda:self.select_file_path(1))
self.dst_path_btn1.grid(row=0, column=1, padx=5, pady=5)
# 添加“选择源路径2”按钮
self.src_path_btn2 = tk.Button(self.root, text="选择源路径2", command=lambda:self.select_file_path(2))
self.src_path_btn2.grid(row=1, column=0, padx=5, pady=5)
# 添加“选择目标路径2”按钮
self.dst_path_btn2 = tk.Button(self.root, text="选择目标路径2", command=lambda:self.select_file_path(3))
self.dst_path_btn2.grid(row=1, column=1, padx=5, pady=5)
# 添加“选择源路径3”按钮
self.src_path_btn3 = tk.Button(self.root, text="选择源路径3", command=lambda:self.select_file_path(4))
self.src_path_btn3.grid(row=2, column=0, padx=5, pady=5)
# 添加“选择目标路径3”按钮
self.dst_path_btn3 = tk.Button(self.root, text="选择目标路径3", command=lambda:self.select_file_path(5))
self.dst_path_btn3.grid(row=2, column=1, padx=5, pady=5)
# 添加“选择源路径4”按钮
self.src_path_btn4 = tk.Button(self.root, text="选择源路径4", command=lambda:self.select_file_path(6))
self.src_path_btn4.grid(row=3, column=0, padx=5, pady=5)
# 添加“选择目标路径4”按钮
self.dst_path_btn4 = tk.Button(self.root, text="选择目标路径4", command=lambda:self.select_file_path(7))
self.dst_path_btn4.grid(row=3, column=1, padx=5, pady=5)
# 添加“选择源路径5”按钮
self.src_path_btn5 = tk.Button(self.root, text="选择源路径5", command=lambda:self.select_file_path(8))
self.src_path_btn5.grid(row=4, column=0, padx=5, pady=5)
# 添加“选择目标路径5”按钮
self.dst_path_btn5 = tk.Button(self.root, text="选择目标路径5", command=lambda:self.select_file_path(9))
self.dst_path_btn5.grid(row=4, column=1, padx=5, pady=5)
# 添加“开始执行”按钮
self.start_btn = tk.Button(self.root, text="开始执行", command=self.start_copy)
self.start_btn.grid(row=5, column=0, padx=5, pady=5)
# 添加“取消执行”按钮
self.cancel_btn = tk.Button(self.root, text="取消执行", command=self.cancel_copy, state=tk.DISABLED)
self.cancel_btn.grid(row=5, column=1, padx=5, pady=5)
def select_file_path(self, index):
# 选择文件路径
file_path = tk.filedialog.askdirectory(initialdir="./")
# 显示选择的文件路径
self.file_path_var[index].set(file_path)
def start_copy(self):
# 禁用“开始执行”按钮
self.start_btn.config(state=tk.DISABLED)
# 启用“取消执行”按钮
self.cancel_btn.config(state=tk.NORMAL)
# 创建1000个线程进行文件复制
for i in range(1000):
for j in range(0, 10, 2):
src_path = self.file_path_var[j].get()
dst_path = self.file_path_var[j+1].get()
if src_path and dst_path:
thread = FileCopyThread(src_path, dst_path)
thread.start()
self.thread_list.append(thread)
# 每隔1秒钟检查一次是否有新文件,如果有则进行复制
self.check_new_file()
def cancel_copy(self):
# 停止所有线程
for thread in self.thread_list:
thread.stop()
# 启用“开始执行”按钮
self.start_btn.config(state=tk.NORMAL)
# 禁用“取消执行”按钮
self.cancel_btn.config(state=tk.DISABLED)
def check_new_file(self):
# 检查源路径中是否有新文件
for i in range(0, 10, 2):
src_path = self.file_path_var[i].get()
dst_path = self.file_path_var[i+1].get()
if src_path and dst_path:
for filename in os.listdir(src_path):
src_file_path = os.path.join(src_path, filename)
dst_file_path = os.path.join(dst_path, filename)
if not os.path.exists(dst_file_path):
# 计算源文件的CRC值
with open(src_file_path, 'rb') as f:
src_crc = zlib.crc32(f.read())
# 如果目标路径中不存在同名文件,则进行复制
shutil.copy(src_file_path, dst_path)
else:
# 计算源文件的CRC值
with open(src_file_path, 'rb') as f:
src_crc = zlib.crc32(f.read())
# 计算目标路径中同名文件的CRC值
with open(dst_file_path, 'rb') as f:
dst_crc = zlib.crc32(f.read())
# 如果CRC值不相等,则进行复制
if src_crc != dst_crc:
shutil.copy(src_file_path, dst_path)
# 每隔1秒钟检查一次
self.root.after(1000, self.check_new_file)
def run(self):
# 启动GUI
self.root.mainloop()
if __name__ == "__main__":
root = tk.Tk()
app = App(root)
```
运行程序后,依次点击“选择源路径1”、“选择目标路径1”、“选择源路径2”、“选择目标路径2”、“选择源路径3”、“选择目标路径3”、“选择源路径4”、“选择目标路径4”、“选择源路径5”、“选择目标路径5”按钮,选择需要复制的源路径和目标路径,然后点击“开始执行”按钮即可开始文件复制。如果需要停止文件复制,可以点击“取消执行”按钮。
相关推荐
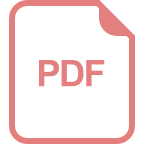
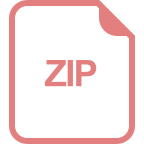
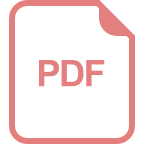
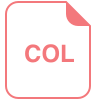













