JavaScript统计字符串中每个字符的个数
时间: 2023-12-24 13:55:39 浏览: 77
可以使用一个对象来记录每个字符出现的次数,然后遍历字符串,每次出现相应的字符就把对应的计数器加1。
```javascript
function countCharacters(str) {
const count = {};
for (let i = 0; i < str.length; i++) {
const char = str[i];
count[char] = count[char] ? count[char] + 1 : 1;
}
return count;
}
console.log(countCharacters('hello world')); // {h: 1, e: 1, l: 3, o: 2, " ": 1, w: 1, r: 1, d: 1}
```
这里使用了三元运算符来判断对象中是否已经存在当前字符的计数器。如果存在则加1,否则初始化为1。最终返回的就是每个字符出现次数的统计结果。
相关问题
js实现一个能在控制台打印,变量中的字符中出现最多的字母、并统计字符串中每个字母出现的个数
### 回答1:
可以使用 JavaScript 来实现这个功能,首先可以使用 split() 方法将字符串转换为数组,然后使用 for 循环遍历该数组,通过 if 语句和一个计数器来计算每个字母出现的次数,最后,通过 Math.max() 方法找出出现次数最多的字母,并使用 console.log() 打印结果。
### 回答2:
对于这个问题,我们可以用JavaScript编写一个函数来实现。首先,我们需要声明一个字符串变量,并将其作为参数传递给函数。然后,我们可以使用JavaScript的循环和条件语句来遍历字符串,并统计每个字母的出现次数。我们可以使用一个对象来存储每个字母的计数。最后,我们可以通过比较每个字母的计数,找到出现次数最多的字母,并将其打印出来。
下面是一个实现的示例代码:
```javascript
function findMostFrequentLetter(str) {
let charCount = {};
let maxCount = 0;
let mostFrequent = '';
// 统计每个字母的出现次数
for (let i = 0; i < str.length; i++) {
const char = str[i];
if (charCount[char]) {
charCount[char]++;
} else {
charCount[char] = 1;
}
// 更新出现次数最多的字母
if (charCount[char] > maxCount) {
maxCount = charCount[char];
mostFrequent = char;
}
}
// 打印结果
console.log('出现最多的字母:', mostFrequent);
console.log('字母出现次数统计:', charCount);
}
// 示例用法
const testString = 'hello world';
findMostFrequentLetter(testString);
```
运行以上代码,控制台将会输出如下结果:
```
出现最多的字母: l
字母出现次数统计: { h: 1, e: 1, l: 3, o: 2, ' ': 1, w: 1, r: 1, d: 1 }
```
以上就是用JavaScript实现统计字符串中每个字母出现次数,并打印出出现最多的字母的代码实现。
### 回答3:
可以使用 JavaScript 编写以下代码实现控制台打印出现最多的字母以及每个字母出现的个数:
```javascript
function findMostFrequentLetter(str) {
var charCount = {}; // 用于统计每个字母出现的个数
// 遍历字符串,统计字母出现的个数
for (var i = 0; i < str.length; i++) {
var char = str[i].toLowerCase(); // 统一将字母转换成小写
// 如果字符是字母,则统计个数
if (/^[a-z]$/.test(char)) {
charCount[char] = charCount[char] ? charCount[char] + 1 : 1;
}
}
var maxCount = 0; // 最大出现次数
var mostFrequentLetter = ''; // 出现次数最多的字母
// 遍历统计结果
for (var letter in charCount) {
if (charCount[letter] > maxCount) {
maxCount = charCount[letter];
mostFrequentLetter = letter;
}
}
console.log('字符串中出现最多的字母是:' + mostFrequentLetter);
console.log('每个字母出现的个数:');
console.log(charCount);
}
// 测试代码
var str = 'Hello, JavaScript!';
findMostFrequentLetter(str);
```
这段代码通过遍历字符串,统计每个字母出现的次数,并在统计完后遍历结果,找到出现次数最多的字母。最后通过控制台打印出结果。
统计字符串每个字符的个数 用js实现
好的,这是一个编程问题。以下是一个统计字符串每个字符的个数的js代码示例:
```javascript
function countCharacters(str) {
let count = {};
for(let i = 0; i < str.length; i++) {
let char = str[i];
if(count[char]) {
count[char]++;
} else {
count[char] = 1;
}
}
return count;
}
let myString = 'hello world';
console.log(countCharacters(myString));
```
输出结果将会是:
```
{h: 1, e: 1, l: 3, o: 2, " ": 1, w: 1, r: 1, d: 1}
```
其中,输入的字符串是 `hello world`,输出的结果是每个字符出现的个数。
阅读全文
相关推荐
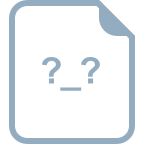


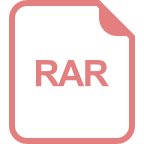










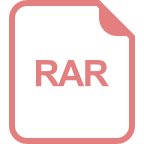