a.c:38:5: error: redefinition of ‘main’ int main() ^~~~ a.c:6:5: note: previous definition of ‘main’ was here int main() ^~~~ a.c: In function ‘main’: a.c:10:2: warning: ignoring return value of ‘scanf’, declared with attribute warn_unused_result [-Wunused-result] scanf("%d%d",&a,&b); ^~~~~~~~~~~~~~~~~~~ a.c: In function ‘main’: a.c:41:5: warning: ignoring return value of ‘scanf’, declared with attribute warn_unused_result [-Wunused-result] scanf("%d%d", &a, &b); ^~~~~~~~~~~~~~~~~~~~~
时间: 2023-07-07 21:45:20 浏览: 76
这个错误提示表明你的代码中有重复定义 main 函数的问题,需要检查一下是否在代码中多次定义了 main 函数。另外,还有两处使用 scanf 函数没有检查其返回值的警告,可以根据需要在代码中进行修改。
以下是修改后的代码,可以参考一下:
```c
#include <stdio.h>
// 求最大公约数
int gcd(int x, int y) {
int r;
while (y != 0) {
r = x % y;
x = y;
y = r;
}
return x;
}
// 求最小公倍数
int lcm(int x, int y) {
return x * y / gcd(x, y);
}
int main() {
int a, b, d, m;
if (scanf("%d%d", &a, &b) != 2) {
printf("Invalid input!\n");
return 0;
}
d = gcd(a, b);
m = lcm(a, b);
printf("Greatest Common Divisor:%d\n", d);
printf("Least_Common_Multiple:%d\n", m);
return 0;
}
```
对于 scanf 函数的返回值检查,可以使用 if 语句对其返回值进行判断,以确保输入的参数个数正确。
相关问题
a.c:26:5: error: redefinition of ‘main’ int main() ^~~~ a.c:14:5: note: previous definition of ‘main’ was here int main() ^~~~ a.c: In function ‘main’: a.c:29:5: warning: ignoring return value of ‘scanf’, declared with attribute warn_unused_result [-Wunused-result] scanf ( "%d", &n ); ^~~~~~~~~~~~~~~~~~
这个错误提示表明在你的代码中定义了多个 `main` 函数,而且前面的 `main` 函数与后面的 `main` 函数定义不一样。这是因为编译器只允许一个 `main` 函数,因为程序的入口只能有一个。
此外,你的代码中还存在一个 `scanf` 函数调用没有检查返回值的警告。这是因为 `scanf` 函数的返回值为成功读取的参数个数,如果返回值不被检查,可能会导致输入错误的结果。
解决方法是:将多余的 `main` 函数删除,同时在 `scanf` 函数调用后检查返回值,如果返回值不是预期的参数个数,则说明输入有误,需要重新输入。以下是修复后的代码示例:
```c
#include <stdio.h>
int reverse(int n) {
int reversed = 0;
while (n > 0) {
reversed = reversed * 10 + n % 10;
n /= 10;
}
return reversed;
}
int main() {
int num;
printf("请输入一个正整数: ");
if (scanf("%d", &num) != 1) {
printf("输入有误,请重新输入。\n");
return 1;
}
printf("逆序数为: %d\n", reverse(num));
return 0;
}
```
注意:在实际开发中,我们应该尽可能地检查所有函数的返回值,以避免潜在的错误。
a.c:22:5: error: redefinition of ‘main’ int main() ^~~~ a.c:14:5: note: previous definition of ‘main’ was here int main() ^~~~ a.c: In function ‘main’: a.c:17:5: warning: ignoring return value of ‘scanf’, declared with attribute warn_unused_result [-Wunused-result] scanf("%d", &num); ^~~~~~~~~~~~~~~~~ a.c: In function ‘main’: a.c:25:5: warning: ignoring return value of ‘scanf’, declared with attribute warn_unused_result [-Wunused-result] scanf ( "%d", &n ); ^~~~~~~~~~~~~~~~~~
这个错误提示表明在你的代码中定义了多个 `main` 函数,而且前面的 `main` 函数与后面的 `main` 函数定义不一样。这是因为编译器只允许一个 `main` 函数,因为程序的入口只能有一个。
此外,你的代码中还存在两个 `scanf` 函数调用没有检查返回值的警告。这是因为 `scanf` 函数的返回值为成功读取的参数个数,如果返回值不被检查,可能会导致输入错误的结果。
解决方法是:将多余的 `main` 函数删除,同时在每个 `scanf` 函数调用后检查返回值,如果返回值不是预期的参数个数,则说明输入有误,需要重新输入。以下是修复后的代码示例:
```c
#include <stdio.h>
int reverse(int n) {
int reversed = 0;
while (n > 0) {
reversed = reversed * 10 + n % 10;
n /= 10;
}
return reversed;
}
int main() {
int num;
printf("请输入一个正整数: ");
if (scanf("%d", &num) != 1) {
printf("输入有误,请重新输入。\n");
return 1;
}
printf("逆序数为: %d\n", reverse(num));
return 0;
}
```
注意:在实际开发中,我们应该尽可能地检查所有函数的返回值,以避免潜在的错误。
相关推荐
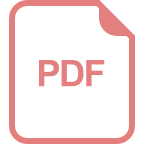
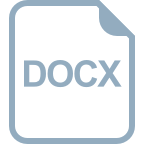
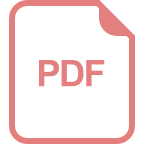









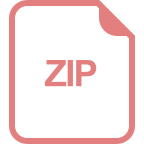
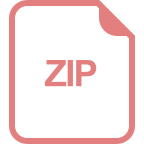
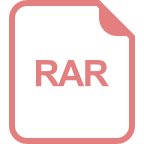