创建mybatis项目,在项目创建Student类及其字段;然后编写代码使用mybatis完成对hytc数据库中的student表中数据的增删改查。
时间: 2024-09-12 22:02:09 浏览: 53
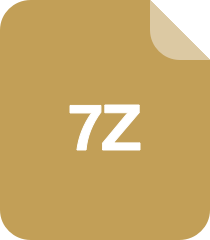
mybatis动态创建数据库表

创建MyBatis项目并实现对hytc数据库中student表的增删改查操作,通常需要以下步骤:
1. 创建项目:首先使用Maven或Gradle等构建工具创建一个Web项目,添加MyBatis和数据库连接池(如c3p0或HikariCP)以及数据库驱动(如MySQL驱动)的依赖。
2. 配置MyBatis:在项目中创建mybatis-config.xml配置文件,配置数据库连接信息,包括数据库URL、用户名、密码、驱动类等。
3. 编写Student类:在Java代码中定义一个Student类,对应数据库中的student表。这个类中应包含与student表列相对应的字段、对应的getter和setter方法。
```java
public class Student {
private Integer id;
private String name;
private Integer age;
private String gender;
// ... 其他字段及getter和setter方法
}
```
4. 创建Mapper接口:定义一个接口(例如StudentMapper.java),在其中定义增删改查等操作的方法签名。
```java
public interface StudentMapper {
int insertStudent(Student student);
int deleteStudentById(Integer id);
int updateStudent(Student student);
Student selectStudentById(Integer id);
List<Student> selectAllStudents();
}
```
5. 编写Mapper XML文件:为上述接口编写对应的Mapper XML文件(例如StudentMapper.xml),在文件中编写SQL语句,并与接口中的方法相对应。
```xml
<mapper namespace="com.example.mapper.StudentMapper">
<insert id="insertStudent" parameterType="Student">
INSERT INTO student (name, age, gender)
VALUES (#{name}, #{age}, #{gender})
</insert>
<!-- 其他增删改查操作的SQL语句 -->
</mapper>
```
6. 配置MyBatis与Mapper:在mybatis-config.xml中注册Mapper XML文件,以便MyBatis能够扫描到并加载这些映射文件。
```xml
<mappers>
<mapper resource="mappers/StudentMapper.xml"/>
</mappers>
```
7. 使用SqlSessionFactory和SqlSession:编写代码创建SqlSessionFactory和SqlSession实例,通过SqlSession执行映射文件中定义的SQL语句,完成数据操作。
```java
try (SqlSession session = sqlSessionFactory.openSession()) {
StudentMapper mapper = session.getMapper(StudentMapper.class);
// 调用mapper接口中的方法执行数据库操作
mapper.insertStudent(new Student());
session.commit();
}
```
8. 处理事务:确保数据库操作的事务性,一般在方法执行完毕后调用session.commit()提交事务,如果操作失败则调用session.rollback()回滚事务。
阅读全文
相关推荐
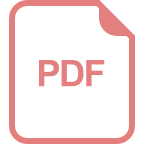
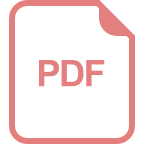
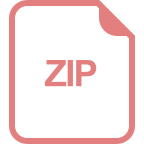
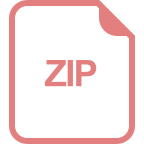
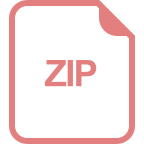
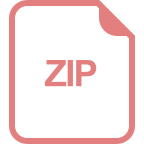
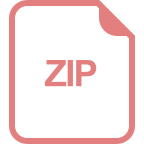
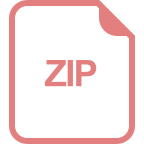
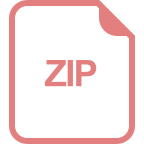
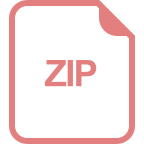
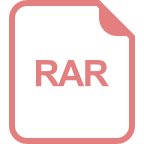
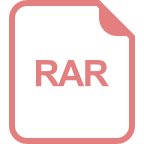
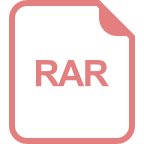
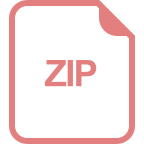
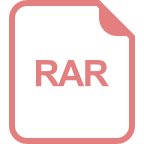
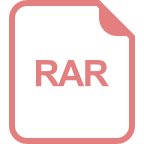
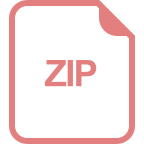
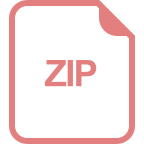