如何用C语言设计一个算法,以保持字符原有的顺序,同时删除字符串S中的所有重复字符?
时间: 2024-10-24 18:08:51 浏览: 26
为了用C语言设计一个算法来保持字符原有的顺序并删除重复字符,你可以使用哈希表(如数组或动态数据结构)来跟踪每个字符是否已经出现过。下面是一个简单的示例,使用`strtok()`函数将字符串分解成单个字符,并用`unordered_set`(无序集合,类似于哈希集)存储已见字符。
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <stdbool.h>
#include <unordered_set>
// 使用哈希表存储字符
typedef struct {
char ch;
bool seen;
} CharNode;
void removeDuplicates(char *s) {
// 初始化哈希表
CharNode *hashTable = (CharNode *)malloc(strlen(s) + 1);
for (int i = 0; i <= strlen(s); i++) {
hashTable[i].ch = '\0';
hashTable[i].seen = false;
}
// 分解字符串,跳过空格
char *token = strtok(s, " ");
while (token != NULL) {
if (!hashTable[strtol(token, NULL, 10)].seen) {
printf("%c", token[0]);
hashTable[strtol(token, NULL, 10)].seen = true;
}
token = strtok(NULL, " ");
}
free(hashTable);
}
int main() {
char s[] = "hello world hello";
removeDuplicates(s);
printf("\n");
return 0;
}
```
在这个例子中,`removeDuplicates` 函数会遍历输入字符串`s`,每次遇到一个非空格字符,它都会检查该字符是否已经在哈希表中。如果未见过,则打印出来并将`seen`标记设置为true。当处理完整个字符串后,就得到了一个新字符串,其中包含了原始顺序但去除了重复的字符。
阅读全文
相关推荐





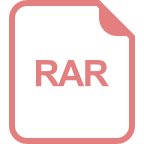

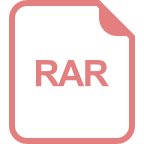

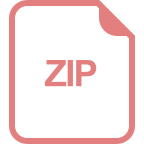



