用Java编写一个程序,实现选择排序法,是:首先找到数组中的最值,将最小值(最大值)的下标与第一个元素的下标交换,再在剩下的元素中找最小值(最大值)的下标与第二个元素的下标交换,完成所有元素的排序
时间: 2024-09-16 19:05:02 浏览: 49
在Java中,我们可以使用一个简单的for循环嵌套结构来实现选择排序算法。这是一个递增版本的选择排序示例,适用于整数数组:
```java
public class SelectionSort {
void selectionSort(int arr[]) {
int n = arr.length;
// 遍历所有数组元素
for (int i = 0; i < n - 1; i++) {
// 找到剩余部分中的最小元素的索引
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
// 将找到的最小元素与当前未排序部分的第一个元素交换位置
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
}
}
// 测试函数
public static void main(String[] args) {
int[] arr = {64, 25, 12, 22, 11};
SelectionSort sort = new SelectionSort();
sort.selectionSort(arr);
System.out.println("Sorted array:");
for (int value : arr) {
System.out.print(value + " ");
}
}
}
```
在这个程序中,`selectionSort`方法首先遍历整个数组,然后在一个内部循环中找到未排序部分的最小值。当外部循环完成后,整个数组就被排序了。
阅读全文
相关推荐
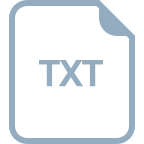
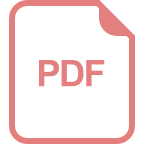
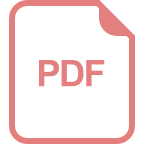

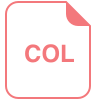


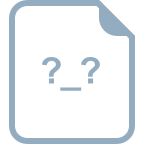
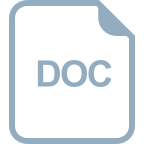
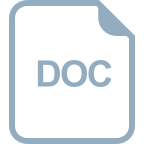
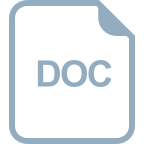
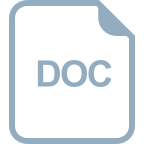
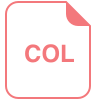
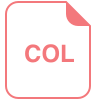
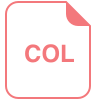



