用C语言编写一个PID控制温度的程序
时间: 2024-12-04 11:29:57 浏览: 20
在C语言中编写PID控制温度的例子通常涉及以下几个步骤:
首先,你需要定义一些全局变量来存储PID参数(比例、积分和微分常数)、当前温度读数、上一次温度和误差等信息。然后,你可以创建一个函数来更新PID控制器的状态,并根据实际温度调整输出信号(例如,风扇速度或加热器功率)。
```c
#include <stdio.h>
#include <time.h>
#define SETPOINT 50 // 设定温度点
#define KP 0.5 // 比例增益
#define KD 0.1 // 微分增益
#define KI 0.01 // 积分增益
#define MAX_OUTPUT 100 // 输出限制
// PID控制器结构体
typedef struct {
float error;
float integral;
float derivative;
float output;
} pid_controller;
void update_pid(pid_controller* pid, float current_temp) {
pid->error = SETPOINT - current_temp; // 当前误差
pid->integral += pid->error * DELTA_TIME; // 积分
pid->derivative = (pid->error - previous_error) / DELTA_TIME; // 微分
pid->output = KP * pid->error + KI * pid->integral + KD * pid->derivative;
// 如果输出超出范围,进行限幅处理
if (pid->output > MAX_OUTPUT)
pid->output = MAX_OUTPUT;
else if (pid->output < -MAX_OUTPUT)
pid->output = -MAX_OUTPUT;
previous_error = pid->error; // 更新上次误差
}
int main() {
pid_controller pid;
float current_temp = 48; // 初始化温度
time_t prev_time = time(NULL);
float DELTA_TIME = 1.0; // 时间间隔(这里假设每秒更新)
while (1) {
time_t curr_time = time(NULL);
DELTA_TIME = difftime(curr_time, prev_time) / CLOCKS_PER_SEC;
update_pid(&pid, current_temp);
printf("Current Temperature: %f, PID Output: %f\n", current_temp, pid.output);
// 这里模拟外部设备对输出信号的反应
current_temp += pid.output;
// ...其他硬件操作...
prev_time = curr_time;
}
return 0;
}
```
注意,这个例子是一个基本的框架,实际应用中还需要考虑温度传感器数据的获取、延时处理和更复杂的中断机制等。此外,PID参数可能需要根据实际情况不断调整优化。
阅读全文
相关推荐
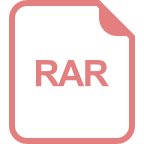
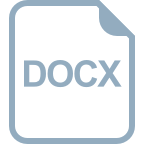
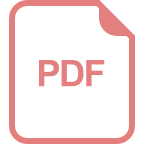

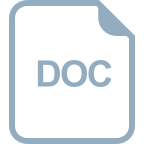
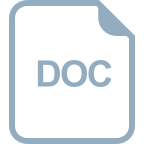


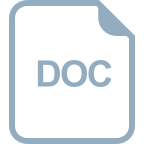
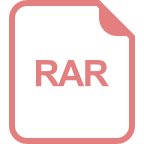
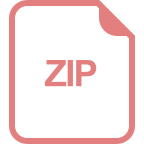
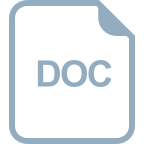
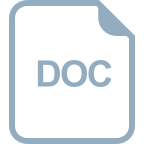
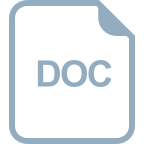
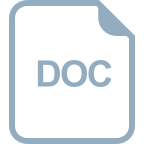
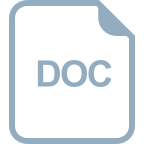
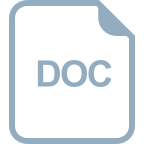

