用C语言编写:1.定义一个带参数的函数,传递一个成绩数组和长度,调用函数输出最高成绩,最低成绩,平均成绩
时间: 2024-12-11 09:19:26 浏览: 13
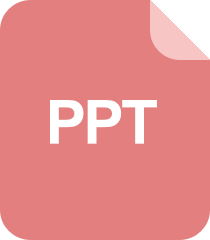
/*定义large函数*/-C语言数组课件学习
在C语言中,你可以定义一个函数来计算数组中的最高、最低成绩以及平均成绩。这里是一个示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义结构体存储单个成绩
typedef struct {
int score;
} Score;
// 函数声明,接受整数数组和其长度
void calculate_scores(int scores[], int length);
// 函数实现
void calculate_scores(int scores[], int length) {
// 初始化最高分和最低分为第一个元素
int max_score = scores[0];
int min_score = scores[0];
// 遍历数组更新最高分和最低分
for (int i = 1; i < length; i++) {
if (scores[i] > max_score) {
max_score = scores[i];
}
if (scores[i] < min_score) {
min_score = scores[i];
}
}
// 计算平均成绩
double average = (double)sum(scores, length) / length;
// 输出结果
printf("最高成绩: %d\n", max_score);
printf("最低成绩: %d\n", min_score);
printf("平均成绩: %.2f\n", average);
// 辅助函数,计算数组总和
int sum(int arr[], int n) {
int total = 0;
for (int i = 0; i < n; i++) {
total += arr[i];
}
return total;
}
}
int main() {
// 创建一个成绩数组
int scores[] = {85, 92, 78, 96, 88};
int length = sizeof(scores) / sizeof(scores[0]);
// 调用函数
calculate_scores(scores, length);
return 0;
}
```
在这个示例中,我们首先定义了一个`Score`结构体来表示单个成绩。然后,`calculate_scores`函数接受一个成绩数组和它的长度,内部通过循环遍历数组找到最高分和最低分,并计算平均值。`sum`是一个辅助函数用于计算数组总和。
要在main函数中测试这个函数,你需要创建一个包含一些成绩的数组并将其传入`calculate_scores`函数。
阅读全文
相关推荐
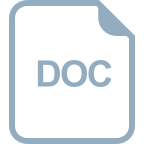
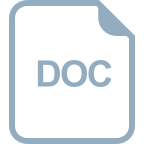
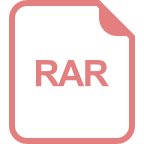
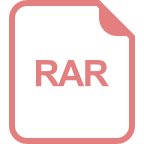
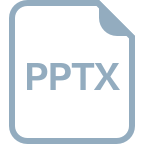
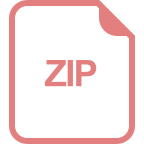
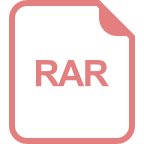
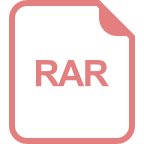
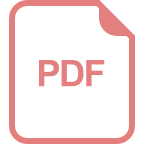
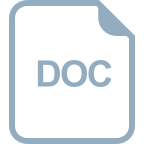
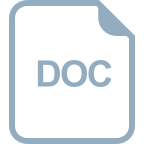
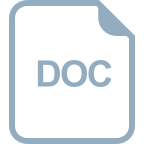
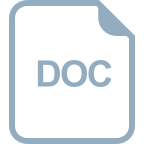
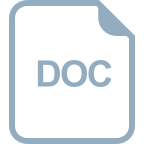
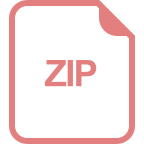
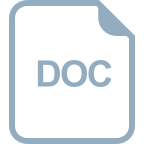