python程序Elasticsearch查看有哪些index
时间: 2024-02-29 10:15:42 浏览: 335
你可以使用Elasticsearch的Python客户端库来获取当前存在的所有索引。以下是一个示例代码:
```python
from elasticsearch import Elasticsearch
# 创建Elasticsearch客户端
es = Elasticsearch()
# 获取所有索引
indices = es.indices.get_alias("*")
# 打印索引列表
for index in indices:
print(index)
```
这段代码首先创建了一个Elasticsearch客户端实例,然后使用`indices.get_alias()`方法来获取所有索引的别名。最后,通过遍历索引列表,你可以打印出所有的索引名称。确保在运行之前安装了`elasticsearch`库,可以使用`pip install elasticsearch`进行安装。
相关问题
python程序elasticsearch查询所有索引
要在Python程序中查询所有的Elasticsearch索引,您可以使用`indices.get_alias` API,并将通配符`*`作为别名参数传递给该API。这将返回与所有索引相关联的别名信息。
以下是一个示例代码:
```python
from elasticsearch import Elasticsearch
# 创建 Elasticsearch 客户端
client = Elasticsearch()
# 查询所有索引
response = client.indices.get_alias(name='*')
# 打印结果
for index in response:
print(index)
```
这段代码将遍历返回的结果,并打印每个索引的名称。
请确保已经安装了`elasticsearch` Python模块,并在代码中正确配置了Elasticsearch客户端的连接信息。
希望这对您有所帮助!如果您还有其他问题,请随时提问。
python es.search
Django是一个由Python编程语言驱动的开源Web应用程序框架,采用了模型-视图-控制器(MVC)的设计模式。使用Django,您可以快速创建高质量、易于维护且与数据库集成的应用程序。Django的核心组件包括模型(Model)、视图(View)和模板(Template)。
在Elasticsearch中,您可以使用bool查询关系来进行复杂的查询。bool查询关系有三种类型:must(必须满足)、should(其中一个满足)和must_not(都不满足)。以下是一些使用bool查询关系的示例:
1. 匹配name为"python"且age为18的所有数据:
```python
body = {
"query": {
"bool": {
"must": [
{ "term": { "name": "python" } },
{ "term": { "age": 18 } }
]
}
}
}
es.search(index="my_index", doc_type="test_type", body=body)
```
2. 切片式查询,从第二条数据开始获取4条数据:
```python
body = {
"query": {
"match_all": {}
},
"from": 2, # 从第二条数据开始
"size": 4 # 获取4条数据
}
es.search(index="my_index", doc_type="test_type", body=body)
```
3. 范围查询,查询age在18到30之间的所有数据:
```python
body = {
"query": {
"range": {
"age": {
"gte": 18, # >=18
"lte": 30 # <=30
}
}
}
}
es.search(index="my_index", doc_type="test_type", body=body)
```
4. 前缀查询,查询name以"p"开头的所有数据:
```python
body = {
"query": {
"prefix": {
"name": "p"
}
}
}
es.search(index="my_index", doc_type="test_type", body=body)
```
5. 通配符查询,查询name以"id"结尾的所有数据:
```python
body = {
"query": {
"wildcard": {
"name": "*id"
}
}
}
es.search(index="my_index", doc_type="test_type", body=body)
```
6. 排序,根据age字段升序排序:
```python
body = {
"query": {
"match_all": {}
},
"sort": {
"age": {
"order": "asc" # asc升序,desc降序
}
}
}
es.search(index="my_index", doc_type="test_type", body=body)
```
您可以在https://elasticsearch-py.readthedocs.io/en/master/api.html上找到更多关于Elasticsearch搜索的用法。
阅读全文
相关推荐
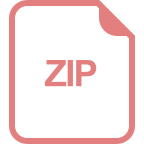
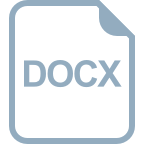
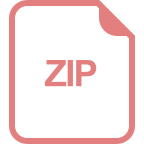
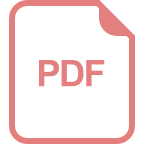
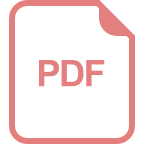
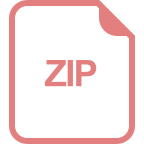
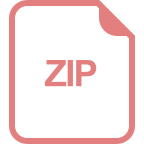
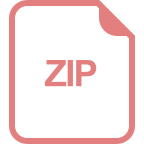
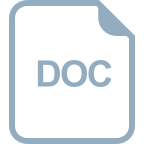
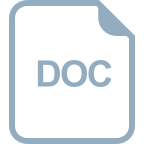
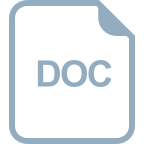
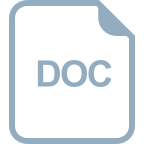
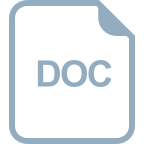
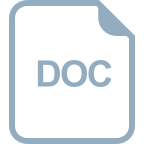
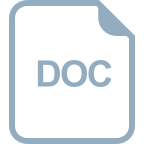
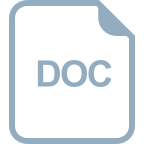