分析一下下列代码public boolean importExcelForIntermediateTable(AuditIntermediateTable intermediateTable, MultipartFile file) { boolean isErrorCondition = intermediateTable == null || StringUtil.isEmpty(intermediateTable.getResourceCategoryId()) || StringUtil.isEmpty(intermediateTable.getTableCategoryId()); if (isErrorCondition) { throw new ValidateException("数据格式异常,请重试!"); } if (StringUtil.isEmpty(intermediateTable.getDbId())) { throw new ValidateException("请选择数据源!"); } BaseDapDb baseDapDb = dapDbMasterService.getById(intermediateTable.getDbId()); if (!file.isEmpty()) { log.info("开始解析Excel"); long startTime = com.huishen.dap.core.util.DateUtil.getCurrentTimeMillis(); try { EasyExcel.read(file.getInputStream(), new IntermediateTableExcelDataListener(baseDapDb, intermediateTable, dapTableService, dapDbMasterService, dynamicTableProperties, this,batchCount)) .sheet().doRead(); } catch (IOException e) { throw new ValidateException("读取Excel数据失败:{}!" + e.getMessage()); } long endTime = com.huishen.dap.core.util.DateUtil.getCurrentTimeMillis(); log.info("Excel解析完成,累计耗时{}s", (endTime - startTime) / 1000.0); return true; } log.error("方法importExcelForColumnValue没有检测到传入的Excel文件!"); return false; }
时间: 2024-02-14 14:29:59 浏览: 100
这段代码是用于导入Excel文件并解析其中的数据。下面是对代码的分析:
1. 首先,代码检查了输入参数`intermediateTable`是否为空,以及其中的关键字段是否为空。如果满足这些错误条件,将抛出`ValidateException`异常,提示数据格式异常。
2. 接下来,代码检查`intermediateTable`中的`dbId`字段是否为空。如果为空,将抛出`ValidateException`异常,提示请选择数据源。
3. 通过`dbId`获取相应的数据库配置信息。
4. 如果传入的文件`file`不为空,开始解析Excel文件。代码使用EasyExcel库读取Excel数据,并通过`IntermediateTableExcelDataListener`处理解析后的数据。
5. 如果读取Excel数据失败,将抛出`ValidateException`异常,提示读取Excel数据失败。
6. 在解析完成后,记录耗时,并返回`true`表示导入成功。
7. 如果传入的文件为空,记录错误日志并返回`false`表示没有检测到传入的Excel文件。
需要注意的是,代码中使用了日志记录来跟踪导入操作的进程和结果。
这段代码整体上看起来逻辑清晰,对异常情况进行了处理。但需要确保`IntermediateTableExcelDataListener`类和相关服务类的实现是正确的,并能正确处理解析后的数据。另外,代码中使用了一些自定义的工具类和方法,需要确保它们的正确性和可用性。
相关问题
<h1>上传/下载文件</h1> <form method="post" action="/file/upload" enctype="multipart/form-data"> <input type="file" name="file" id="fileInput"/> <input type="submit" value="上传" /> </form> <hr> <form method="post" action="/file/download" > <input type="text" name="fileName" placeholder="请输入文件名" /> <input type="submit" value="下载" id="downloadBtn" /> </form> public class SFTPUtil { private static final String host = "10.60.0.160"; private static final int port = 22; private static final String username = "root"; private static final String password = "Z20"; private static Session session = null; public static void connect() throws Exception { } public static void upload(MultipartFile file, String path, String fileName) throws Exception { } public static boolean download(HttpServletResponse response, String path, String fileName) throws Exception { } @Controller @RequestMapping("/file") public class FileController { private static final String UPLOAD_PATH = "/data/sftp/adtuser1/upload/winbidding/"; private static final String DOWNLOAD_PATH = "/data/sftp/adtuser1/upload/winbidding/"; @PostMapping("/upload") @ResponseBody public ModelAndView upload(@RequestParam("file") MultipartFile file) { } @PostMapping("/download") public ModelAndView download(@RequestParam("fileName") String fileName, HttpServletResponse response) throws Exception { } 数据库有host port username password UPLOAD_PATH DOWNLOAD_PATH csmc字段,把上边写死的改成数据库查询,前台页面增加csmc字段下拉框,根据csmc字段把查出来的配置数据完成上传下载功能,用的mysql数据库,把代码改好写出来
首先需要在数据库中创建一个表,用于存储SFTP连接的相关信息:
```sql
CREATE TABLE sftp_config (
id INT PRIMARY KEY AUTO_INCREMENT,
csmc VARCHAR(50),
host VARCHAR(50),
port INT,
username VARCHAR(50),
password VARCHAR(50),
upload_path VARCHAR(255),
download_path VARCHAR(255)
);
```
然后在Spring Boot项目中创建一个SFTPUtil类,用于实现SFTP上传和下载功能:
```java
import com.jcraft.jsch.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
import java.util.Properties;
public class SFTPUtil {
private String host;
private int port;
private String username;
private String password;
private String uploadPath;
private String downloadPath;
private Session session;
public SFTPUtil(String host, int port, String username, String password, String uploadPath, String downloadPath) {
this.host = host;
this.port = port;
this.username = username;
this.password = password;
this.uploadPath = uploadPath;
this.downloadPath = downloadPath;
}
public void connect() throws Exception {
JSch jsch = new JSch();
session = jsch.getSession(username, host, port);
session.setPassword(password);
Properties config = new Properties();
config.put("StrictHostKeyChecking", "no");
session.setConfig(config);
session.connect();
}
public void upload(MultipartFile file, String fileName) throws Exception {
ChannelSftp channel = (ChannelSftp) session.openChannel("sftp");
channel.connect();
channel.cd(uploadPath);
InputStream inputStream = file.getInputStream();
channel.put(inputStream, fileName);
inputStream.close();
channel.disconnect();
}
public boolean download(HttpServletResponse response, String fileName) throws Exception {
ChannelSftp channel = (ChannelSftp) session.openChannel("sftp");
channel.connect();
channel.cd(downloadPath);
SftpATTRS attrs = channel.lstat(fileName);
if (attrs == null) {
channel.disconnect();
return false;
}
response.setHeader("Content-Disposition", "attachment; filename=\"" + fileName + "\"");
OutputStream outputStream = response.getOutputStream();
channel.get(fileName, outputStream);
outputStream.flush();
outputStream.close();
channel.disconnect();
return true;
}
}
```
然后在Spring Boot项目中创建一个FileController类,用于处理文件上传和下载请求:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletResponse;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/file")
public class FileController {
@Autowired
private JdbcTemplate jdbcTemplate;
@PostMapping("/upload")
@ResponseBody
public String upload(@RequestParam("file") MultipartFile file, @RequestParam("csmc") String csmc) {
try {
// 从数据库中获取SFTP连接配置信息
String sql = "SELECT * FROM sftp_config WHERE csmc = ?";
Map<String, Object> map = jdbcTemplate.queryForMap(sql, csmc);
String host = (String) map.get("host");
int port = (int) map.get("port");
String username = (String) map.get("username");
String password = (String) map.get("password");
String uploadPath = (String) map.get("upload_path");
String fileName = file.getOriginalFilename();
// 使用SFTP上传文件
SFTPUtil sftpUtil = new SFTPUtil(host, port, username, password, uploadPath, null);
sftpUtil.connect();
sftpUtil.upload(file, fileName);
return "上传成功";
} catch (Exception e) {
e.printStackTrace();
return "上传失败";
}
}
@PostMapping("/download")
public void download(@RequestParam("fileName") String fileName, @RequestParam("csmc") String csmc, HttpServletResponse response) throws Exception {
// 从数据库中获取SFTP连接配置信息
String sql = "SELECT * FROM sftp_config WHERE csmc = ?";
Map<String, Object> map = jdbcTemplate.queryForMap(sql, csmc);
String host = (String) map.get("host");
int port = (int) map.get("port");
String username = (String) map.get("username");
String password = (String) map.get("password");
String downloadPath = (String) map.get("download_path");
// 使用SFTP下载文件
SFTPUtil sftpUtil = new SFTPUtil(host, port, username, password, null, downloadPath);
sftpUtil.connect();
boolean result = sftpUtil.download(response, fileName);
if (!result) {
response.getWriter().write("文件不存在");
}
}
@GetMapping("/csmcList")
@ResponseBody
public List<String> getCsmcList() {
// 从数据库中获取所有的csmc字段
String sql = "SELECT DISTINCT csmc FROM sftp_config";
List<String> csmcList = jdbcTemplate.queryForList(sql, String.class);
return csmcList;
}
}
```
最后,在HTML页面中加入下面的代码,即可实现文件上传和下载:
```html
<h1>上传/下载文件</h1>
<form method="post" action="/file/upload" enctype="multipart/form-data">
<input type="file" name="file" id="fileInput"/>
<select name="csmc" id="csmcSelect"></select>
<input type="submit" value="上传"/>
</form>
<hr>
<form method="post" action="/file/download">
<input type="text" name="fileName" placeholder="请输入文件名"/>
<select name="csmc" id="csmcSelect"></select>
<input type="submit" value="下载" id="downloadBtn"/>
</form>
<script>
// 获取csmc列表
$.get("/file/csmcList", function (data) {
var csmcSelect = $("#csmcSelect");
for (var i = 0; i < data.length; i++) {
var option = $("<option>").val(data[i]).text(data[i]);
csmcSelect.append(option);
}
});
</script>
```
改进这段代码: public R<Void> importData(@RequestPart("file") MultipartFile file, boolean updateSupport) throws Exception { ExcelResult<SysUserImportVo> result = ExcelUtil.importExcel(file.getInputStream(), SysUserImportVo.class, new SysUserImportListener(updateSupport)); return R.ok(result.getAnalysis()); }
可以尝试以下改进方案:
1. 增加异常处理:在方法声明中已经声明了抛出 Exception,但是在方法内部并没有对异常进行处理。可以使用 try-catch 块来捕获异常,或者在方法声明中更明确地声明具体的异常类型。
2. 增加日志记录:在方法执行过程中,可以记录一些关键的信息,例如文件名、导入结果等,以便出现问题时快速定位问题。
3. 增加参数校验:对于文件上传这种操作,需要进行一些基本的参数校验,例如文件大小、文件类型等,以避免上传不合法的文件。
4. 返回结果优化:当前方法返回类型为 R<Void>,虽然可以通过 getAnalysis() 方法获取导入结果,但是需要额外的操作。可以考虑直接返回导入结果,或者将 R<Void> 改为 R<ExcelResult<SysUserImportVo>>。
改进后的代码如下所示:
```
public R<ExcelResult<SysUserImportVo>> importData(@RequestPart("file") MultipartFile file, boolean updateSupport) throws IOException {
// 参数校验
if (file == null || file.isEmpty()) {
return R.error("文件不能为空");
}
if (file.getSize() > MAX_FILE_SIZE) {
return R.error("文件大小不能超过" + MAX_FILE_SIZE / 1024 / 1024 + "MB");
}
String fileName = file.getOriginalFilename();
if (!fileName.endsWith(".xls") && !fileName.endsWith(".xlsx")) {
return R.error("文件格式不正确,只支持 Excel 文件");
}
// 执行导入操作
try {
ExcelResult<SysUserImportVo> result = ExcelUtil.importExcel(file.getInputStream(), SysUserImportVo.class, new SysUserImportListener(updateSupport));
// 记录导入日志
log.info("文件{}导入成功,共导入{}条数据", fileName, result.getTotalCount());
return R.ok(result);
} catch (Exception e) {
// 记录导入日志
log.error("文件{}导入失败:{}", fileName, e.getMessage());
throw new RuntimeException("文件导入失败,请检查文件格式或内容是否正确");
}
}
```
阅读全文
相关推荐
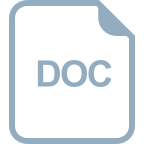
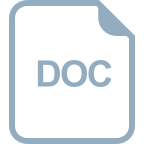
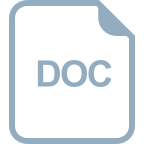













