创建一个职工类Emplyee,其属性有员工编号id、姓名name、性别sex、薪水 salary;再声明三个职工对象并赋值,然后依次使用List、Set、Map集合来实现对职工对象数据的存储、添加、删除、修改、查询等操作,并输出。
时间: 2024-11-06 18:22:33 浏览: 14
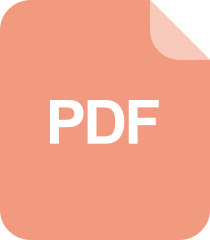
Java 上机作业2020.5.8
首先,我们创建一个名为`Employee`的简单Java类,代表职工:
```java
public class Employee {
private int id;
private String name;
private String sex;
private double salary;
// 构造函数
public Employee(int id, String name, String sex, double salary) {
this.id = id;
this.name = name;
this.sex = sex;
this.salary = salary;
}
// 获取和设置属性的方法
public int getId() { return id; }
public void setId(int id) { this.id = id; }
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public String getSex() { return sex; }
public void setSex(String sex) { this.sex = sex; }
public double getSalary() { return salary; }
public void setSalary(double salary) { this.salary = salary; }
@Override
public String toString() {
return "Employee{" +
"id=" + id +
", name='" + name + '\'' +
", sex='" + sex + '\'' +
", salary=" + salary +
'}';
}
}
```
接下来,我们可以创建三个职工对象并演示如何使用不同的集合结构进行存储:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
// 创建员工对象
Employee emp1 = new Employee(1, "张三", "男", 5000);
Employee emp2 = new Employee(2, "李四", "女", 6000);
Employee emp3 = new Employee(3, "王五", "男", 7000);
// 使用List存储
List<Employee> list = new ArrayList<>();
list.add(emp1);
list.add(emp2);
list.add(emp3);
System.out.println("List: " + list);
// 使用Set(无重复元素)
Set<Employee> set = new HashSet<>(list);
System.out.println("Set (unique): " + set);
// 使用Map存储(key-value形式)
Map<Integer, Employee> map = new HashMap<>();
map.put(emp1.getId(), emp1);
map.put(emp2.getId(), emp2);
map.put(emp3.getId(), emp3);
System.out.println("Map: " + map);
// 删除、修改和查询操作示例
list.remove(emp1); // 删除emp1
emp2.setName("李四新"); // 修改emp2的姓名
System.out.println("After remove and modify:");
System.out.println("List after removing emp1: " + list);
System.out.println("Modified emp2: " + map.get(map.keySet().iterator().next()));
// 查询操作(假设id作为键)
Employee queryEmp = map.get(2); // 查找id为2的员工
if (queryEmp != null) {
System.out.println("Found employee with id 2: " + queryEmp);
} else {
System.out.println("Employee not found.");
}
}
}
```
阅读全文
相关推荐
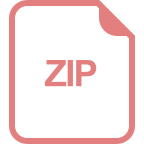
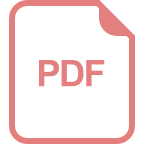

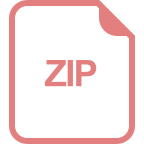
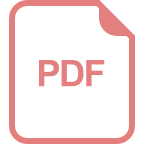
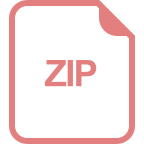
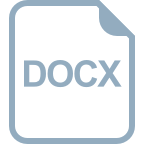
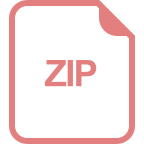
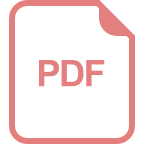
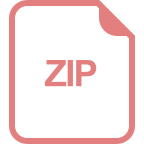