ffmpeg 视频合并 c代码 不使用system系统命令 要调用ffmpeg的api
时间: 2023-08-27 20:52:08 浏览: 160
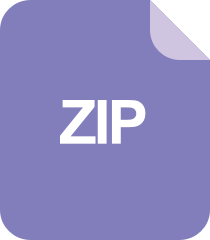
C#ffmpeg调用命令.zip
如果你想使用FFmpeg的API来进行视频合并,你可以按照以下步骤进行。
首先,你需要包含FFmpeg的头文件,并链接FFmpeg库。你可以使用以下代码来实现:
```c
#include <stdio.h>
#include <libavformat/avformat.h>
#include <libavutil/error.h>
int main() {
// 注册所有的FFmpeg组件
av_register_all();
// 打开第一个输入文件
AVFormatContext* input1_ctx = avformat_alloc_context();
if (avformat_open_input(&input1_ctx, "input1.mp4", NULL, NULL) != 0) {
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_strerror(errno, errbuf, sizeof(errbuf));
printf("无法打开输入文件1: %s\n", errbuf);
return -1;
}
// 打开第二个输入文件
AVFormatContext* input2_ctx = avformat_alloc_context();
if (avformat_open_input(&input2_ctx, "input2.mp4", NULL, NULL) != 0) {
char errbuf[AV_ERROR_MAX_STRING_SIZE];
av_strerror(errno, errbuf, sizeof(errbuf));
printf("无法打开输入文件2: %s\n", errbuf);
return -1;
}
// 创建输出上下文
AVFormatContext* output_ctx = avformat_alloc_context();
// 新建输出文件
if (avformat_alloc_output_context2(&output_ctx, NULL, NULL, "output.mp4") < 0) {
printf("无法创建输出文件\n");
return -1;
}
// 遍历输入文件的流
for (int i = 0; i < input1_ctx->nb_streams; i++) {
// 创建输出流
AVStream* output_stream = avformat_new_stream(output_ctx, NULL);
if (!output_stream) {
printf("无法创建输出流\n");
return -1;
}
// 复制输入流的参数到输出流
if (avcodec_parameters_copy(output_stream->codecpar, input1_ctx->streams[i]->codecpar) < 0) {
printf("无法复制流参数\n");
return -1;
}
}
for (int i = 0; i < input2_ctx->nb_streams; i++) {
// 创建输出流
AVStream* output_stream = avformat_new_stream(output_ctx, NULL);
if (!output_stream) {
printf("无法创建输出流\n");
return -1;
}
// 复制输入流的参数到输出流
if (avcodec_parameters_copy(output_stream->codecpar, input2_ctx->streams[i]->codecpar) < 0) {
printf("无法复制流参数\n");
return -1;
}
}
// 打开输出文件
if (!(output_ctx->oformat->flags & AVFMT_NOFILE)) {
if (avio_open(&output_ctx->pb, "output.mp4", AVIO_FLAG_WRITE) < 0) {
printf("无法打开输出文件\n");
return -1;
}
}
// 写入文件头
if (avformat_write_header(output_ctx, NULL) < 0) {
printf("无法写入文件头\n");
return -1;
}
// 读取第一个输入文件的数据并写入输出文件
AVPacket packet;
while (av_read_frame(input1_ctx, &packet) >= 0) {
packet.stream_index = av_find_best_stream(input1_ctx, packet.stream_index, -1, -1, NULL, 0);
av_interleaved_write_frame(output_ctx, &packet);
av_packet_unref(&packet);
}
// 读取第二个输入文件的数据并写入输出文件
while (av_read_frame(input2_ctx, &packet) >= 0) {
packet.stream_index = av_find_best_stream(input2_ctx, packet.stream_index, -1, -1, NULL, 0);
av_interleaved_write_frame(output_ctx, &packet);
av_packet_unref(&packet);
}
// 写入文件尾
av_write_trailer(output_ctx);
// 释放资源
avformat_close_input(&input1_ctx);
avformat_close_input(&input2_ctx);
avformat_free_context(output_ctx);
printf("视频合并成功\n");
return 0;
}
```
上面的代码使用了FFmpeg的API来打开输入文件、创建输出文件、复制流参数、读取数据并写入输出文件等操作。你可以根据自己的需求进行修改和扩展。
请注意,你需要安装并配置好FFmpeg库,并将其包含在你的项目中。另外,记得将输入文件和输出文件的路径替换为你实际的文件路径。
希望对你有所帮助!如果有其他问题,请随时提问。
阅读全文
相关推荐
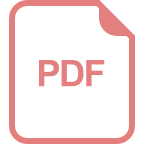
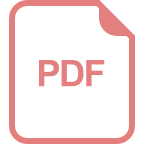










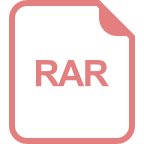
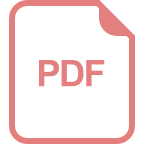
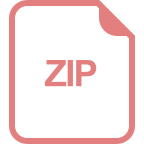
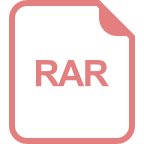
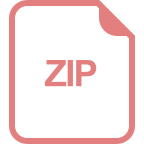
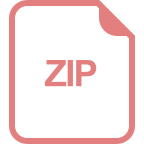