用c#写的WinForm项目如何调用c++程序
时间: 2023-06-17 14:08:40 浏览: 82
在C#中调用C++程序需要使用平台调用(P/Invoke)技术。下面是一些基本的步骤:
1. 将C++程序编译为动态链接库(DLL)文件。
2. 在C#项目中添加对DLL文件的引用。
3. 在C#中声明C++函数的签名。
4. 使用DllImport属性将声明的函数与DLL文件中的函数进行映射。
5. 在C#中调用映射的函数。
下面是一个示例代码:
C++代码:
```
__declspec(dllexport) int Add(int a, int b)
{
return a + b;
}
```
C#代码:
```
using System.Runtime.InteropServices;
class Program
{
[DllImport("MyCppLibrary.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern int Add(int a, int b);
static void Main(string[] args)
{
int result = Add(1, 2);
Console.WriteLine(result);
}
}
```
请注意,调用C++函数时,必须使用正确的参数类型和调用约定(Calling Convention)。在本例中,我们使用了Cdecl调用约定。如果C++函数使用了其他调用约定,需要相应地更改DllImport属性。
相关问题
如何使用vscode写c# winform
使用VS Code写C语言的步骤如下:
1. 安装编译器:在 VS Code 中编写 C 代码需要安装 C 编译器,可选项有 gcc、clang、mingw、tcc 等,建议使用 gcc 或者 mingw,更加稳定和流行。
2. 安装 C/C++ 插件:在 VS Code 中安装 C/C++ 插件,该插件支持代码高亮、代码格式化、错误检查等功能,可大幅提升开发效率。
3. 新建 C 文件:在 VS Code 中新建一个 C 文件,具体操作是在“文件”菜单中选择“新建文件”,然后命名文件以.c为后缀,例如“hello.c”。
4. 编写代码:在新建的 C 文件中编写代码,VS Code 支持代码补全、自动格式化等功能,可以大幅提高编码效率。
5. 编译运行:在 VS Code 中编写完 C 代码后,需要将代码编译成可执行文件。可以在终端中使用 gcc 或者 mingw 编译,也可以使用 VS Code 的内置任务运行器,快捷键是 Ctrl + Shift + B,编译完成后,可在终端中运行生成的可执行文件。
6. 调试程序:在 VS Code 中调试 C 程序也非常方便,可以通过 F5 快捷键进入调试模式,设置断点、单步执行等,进行程序调试。
以上就是在 VS Code 中写 C 的基本步骤,总的来说,使用 VS Code 写 C 语言非常方便,需要的工具和插件也很容易安装,可以大幅提高编程效率和体验。
c# winform调用接口获取token存到缓存
C是计算机科学中的一种编程语言。它是由丹尼斯·里奇和肯·汤普森在20世纪70年代早期开发的,在1972年首次正式发布。C是一种结构化、高级和通用的编程语言,用于开发各种应用程序,包括操作系统、嵌入式系统、网络应用、图形应用等。
C语言具有简洁、高效、灵活的特点,因此在编写底层和系统级的代码中得到广泛应用。C语言提供了丰富的库函数和操作符,使程序员能够更轻松地开发功能丰富的程序。C语言也支持指针操作,这使得程序员可以直接访问和操作内存,提高了程序的执行效率。
C语言的语法简单明了,易于学习和理解。它采用了自顶向下的开发模式,即先定义函数原型,再编写函数体,使得程序结构更加清晰。与其他语言相比,C语言的执行速度更快,占用的内存更小,因此在对性能要求较高的应用场景中得到广泛应用。
C语言还是其他一些编程语言的基础,如C++、Java等。学习和掌握C语言可以为日后学习其他编程语言打下良好的基础。
总之,C语言是一种重要且广泛应用的编程语言,它具有简洁、高效、灵活的特点,被广泛应用于各种应用程序的开发中。
相关推荐
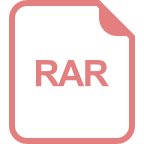












