用python实现在html 上填写信息添加到MySQL
时间: 2024-01-24 08:16:54 浏览: 78
实现这个功能需要以下步骤:
1. 安装 Python 的 MySQL 驱动程序,可以使用 PyMySQL 或者 mysql-connector-python,这里以 PyMySQL 为例。
```
pip install pymysql
```
2. 创建一个 HTML 页面,包含需要填写的表单,例如一个包含用户名和密码的登录表单。
```html
<!DOCTYPE html>
<html>
<body>
<form action="submit.py" method="post">
<label for="username">Username:</label><br>
<input type="text" id="username" name="username"><br>
<label for="password">Password:</label><br>
<input type="password" id="password" name="password"><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
```
3. 创建一个 Python 脚本,用于接收表单提交的数据,并将数据插入到 MySQL 数据库中。例如,创建一个名为 submit.py 的文件。
```python
import pymysql.cursors
import cgi
# 连接 MySQL 数据库
connection = pymysql.connect(host='localhost',
user='root',
password='password',
db='mydatabase',
charset='utf8mb4',
cursorclass=pymysql.cursors.DictCursor)
# 获取表单数据
form = cgi.FieldStorage()
username = form.getvalue('username')
password = form.getvalue('password')
# 将数据插入到 MySQL 数据库中
try:
with connection.cursor() as cursor:
sql = "INSERT INTO `users` (`username`, `password`) VALUES (%s, %s)"
cursor.execute(sql, (username, password))
connection.commit()
finally:
connection.close()
# 返回一个成功提示页面
print("Content-type: text/html\n")
print("<html>")
print("<head>")
print("<title>Submit Success</title>")
print("</head>")
print("<body>")
print("<h2>Submit Success!</h2>")
print("</body>")
print("</html>")
```
4. 将 HTML 文件和 Python 脚本文件放在同一个目录下,并在浏览器中打开 HTML 文件,填写表单并提交,数据将被插入到 MySQL 数据库中。注意,需要确保 MySQL 数据库已经启动,并且数据库和表已经创建。
阅读全文
相关推荐
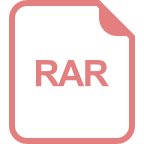
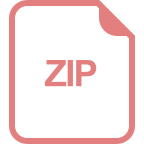
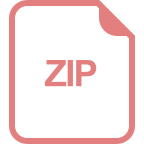












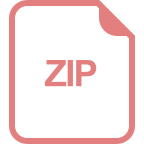
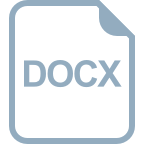
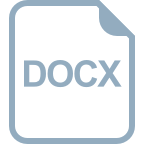
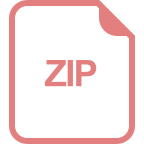