使用unity将base64转为图片
时间: 2023-10-11 13:22:09 浏览: 28
在 Unity 中将 base64 编码的字符串转换为图片可以使用以下代码:
```csharp
using System;
using System.IO;
using UnityEngine;
public static class Base64Utils
{
public static Texture2D Base64ToTexture2D(string base64)
{
string[] base64Parts = base64.Split(',');
if (base64Parts.Length != 2)
{
Debug.LogError("Invalid base64 input string!");
return null;
}
string imageType = base64Parts[0].Replace("data:", "").Replace(";base64", "");
if (imageType != "image/png" && imageType != "image/jpeg")
{
Debug.LogError("Unsupported image type: " + imageType);
return null;
}
Texture2D texture = new Texture2D(1, 1);
byte[] imageData = Convert.FromBase64String(base64Parts[1]);
if (texture.LoadImage(imageData))
{
return texture;
}
Debug.LogError("Failed to load image data!");
return null;
}
}
```
这段代码定义了一个 `Base64Utils` 静态类,其中有一个 `Base64ToTexture2D` 方法可以将 base64 编码的字符串转换为 `Texture2D` 对象。这个方法首先分离出 base64 字符串中的图片类型和编码数据,然后根据图片类型创建一个新的 `Texture2D` 对象,并使用 `Convert.FromBase64String` 方法将 base64 编码的字符串解码为字节数组,最后使用 `texture.LoadImage` 方法将字节数组加载到 `Texture2D` 对象中。
你可以在你的代码中使用该静态方法,例如:
```csharp
string base64Str = "data:image/png;base64,iVBORw0KG...QmCC";
Texture2D texture = Base64Utils.Base64ToTexture2D(base64Str);
if (texture != null)
{
// 将 texture 作为图片显示在 UI 或 3D 对象上
}
```
阅读全文
相关推荐
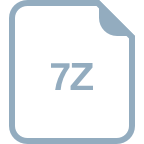
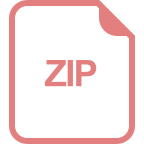
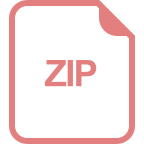
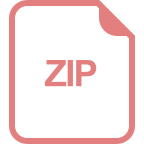
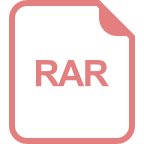
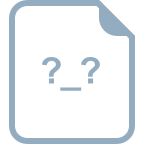
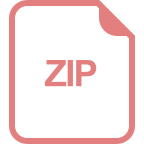
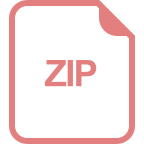
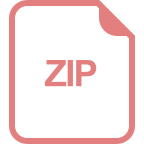
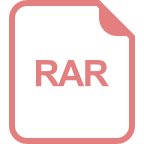