python做一个用户界面
时间: 2024-10-01 09:02:13 浏览: 30
Python可以使用多种库来创建用户界面(UI),以下是几个常用的库:
1. **Tkinter**: Python自带的标准GUI库,它提供了一个简单易学的基础,适合初学者入门。你可以通过创建窗口、标签、按钮等组件来构建基本的图形用户界面。
```python
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text="Hello, World!")
button = tk.Button(root, text="Click me", command=root.quit)
label.pack()
button.pack()
root.mainloop()
```
2. **PyQt/PySide**: 这是基于Qt库的绑定,功能强大且适用于复杂应用。它们提供了丰富的控件和布局系统,以及动画效果支持。
```python
from PyQt5.QtWidgets import QApplication, QWidget, QLabel
app = QApplication([])
window = QWidget()
window.setWindowTitle("My App")
label = QLabel(window, text="Hello Qt!")
layout = QVBoxLayout(window)
layout.addWidget(label)
window.show()
app.exec_()
```
3. **Kivy**: Kivy是一个跨平台的开源框架,特别适合用于创建移动和触摸设备上的用户界面,支持动画和多点触控。
```python
from kivy.app import App
from kivy.uix.button import Button
class MyApp(App):
def build(self):
return Button(text="Hello Kivy!")
if __name__ == "__main__":
MyApp().run()
```
4. **wxPython**: 使用这个库可以在Python中创建类似Windows的应用程序,拥有丰富的控件和定制选项。
```python
import wx
class MyWindow(wx.Frame):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.InitUI()
def InitUI(self):
btn = wx.Button(self, label="Hello wxPython")
btn.Bind(wx.EVT_BUTTON, self.OnButtonClick)
def OnButtonClick(self, event):
print("Button clicked")
if __name__ == "__main__":
app = wx.App()
win = MyWindow(None, title="wxPython UI")
win.Show()
app.MainLoop()
```
阅读全文
相关推荐
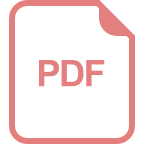
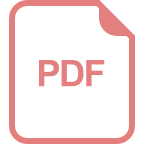















